LifeCycle
In the context of Spring beans (which I believe is the context of what you are reading - hard to tell with the little info you've provided), beans go through different lifecycle phases (like creation and destruction). Here are the lifecycle phases of the Spring bean you can hook into:
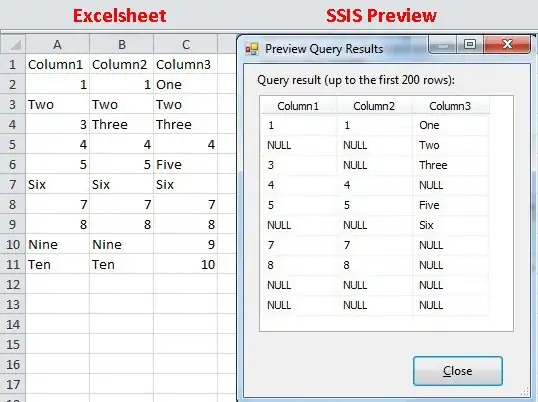
Callback
@R.T.'s wikipedia link to what a callback is, is a good starting point to understanding callbacks. In Java, the concept of callback is implemented differently.
In object-oriented programming languages without function-valued arguments, such as in Java before its 1.7 version, callbacks can be simulated by passing an instance of an abstract class or interface, of which the receiver will call one or more methods, while the calling end provides a concrete implementation.
A good example is given by @SotiriosDelamanolis in this answer, which I'll post here just for context.
/**
* @author @SotiriosDelamanolis
* see https://stackoverflow.com/a/19405498/2587435
*/
public class Test {
public static void main(String[] args) throws Exception {
new Test().doWork(new Callback() { // implementing class
@Override
public void call() {
System.out.println("callback called");
}
});
}
public void doWork(Callback callback) {
System.out.println("doing work");
callback.call();
}
public interface Callback {
void call();
}
}
LifeCycle Callback
By looking at the image above, you can see that Spring allows you to hook into the bean lifecyle with some interfaces and annotations. For example
Hooking into the bean creation part of the lifecycle, you can implements InitializingBean
, which has a callback method afterPropertiesSet()
. When you implements this interface, Spring pick up on it, and calls the afterPropertiesSet()
.
For example
public class SomeBean implements InitializingBean {
@Override
public void afterPropertiesSet() { // this is the callback method
// for the bean creation phase of the
// spring bean lifecycle
// do something after the properties are set during bean creation
}
}
Alternatively, you can use the @PostConstruct
method for a non-InitializingBean implemented method, or using the init-method
in xml config.
The diagram shows other lifecycle phases you can hook into and provide "callback" method for. The lifecycle phases are underlined at the top in the diagram
You can see more at Spring reference - Lifecycle Callbacks