As the examples show, you'll need to put plt.xkcd()
at the start of the code, before all the plotting commands. Thus:
from matplotlib import pyplot as plt
import numpy as np
x = np.arange(10)
y = np.sin(x)
plt.xkcd()
fig = plt.figure()
ax = fig.add_subplot(1,1,1)
ax1 = fig.add_subplot(1,1,1)
ax1.plot(x,y)
ax1.xaxis.set_visible(False)
ax1.yaxis.set_visible(False)
plt.axvline(x=2.3, color='k', ls='dashed')
plt.axvline(x=6, color='k', ls='dashed')
ax.text(4,4,'Culture Shock', size=16)
plt.title('Test title')
plt.show()
which results in this figure for me:
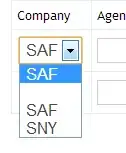
As you see, the wobbly lines are there, but the font is wrong, simply because it's not available on my Linux machine (I also get a warning about this on the command line).
Putting plt.xkcd()
at the end of the code results in the straightforward matplotlib figure, without the wobbly lines.
Here is a summary of what pyplot.xkcd()
does under the hood; it just sets a lot of resource parameters:
rcParams['font.family'] = ['Humor Sans', 'Comic Sans MS']
rcParams['font.size'] = 14.0
rcParams['path.sketch'] = (scale, length, randomness)
rcParams['path.effects'] = [
patheffects.withStroke(linewidth=4, foreground="w")]
rcParams['axes.linewidth'] = 1.5
rcParams['lines.linewidth'] = 2.0
rcParams['figure.facecolor'] = 'white'
rcParams['grid.linewidth'] = 0.0
rcParams['axes.unicode_minus'] = False
rcParams['axes.color_cycle'] = ['b', 'r', 'c', 'm']
rcParams['xtick.major.size'] = 8
rcParams['xtick.major.width'] = 3
rcParams['ytick.major.size'] = 8
rcParams['ytick.major.width'] = 3