To wait for input and to display some random output at the same time, you could use a GUI (something with an event loop):
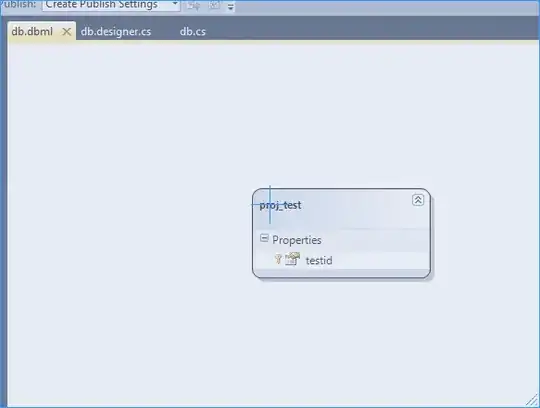
#!/usr/bin/env python3
import random
from tkinter import E, END, N, S, scrolledtext, Tk, ttk, W
class App:
password = "123456" # the most common password
def __init__(self, master):
self.master = master
self.master.title('To stop, type: ' + self.password)
# content frame (padding, etc)
frame = ttk.Frame(master, padding="3 3 3 3")
frame.grid(column=0, row=0, sticky=(N, W, E, S))
# an area where random messages to appear
self.textarea = scrolledtext.ScrolledText(frame)
# an area where the password to be typed
textfield = ttk.Entry(frame)
# put one on top of the other
self.textarea.grid(row=0)
textfield.grid(row=1, sticky=(E, W))
textfield.bind('<KeyRelease>', self.check_password)
textfield.focus() # put cursor into the entry
self.update_textarea()
def update_textarea(self):
# insert random Unicode codepoint in U+0000-U+FFFF range
character = chr(random.choice(range(0xffff)))
self.textarea.configure(state='normal') # enable insert
self.textarea.insert(END, character)
self.textarea.configure(state='disabled') # disable editing
self.master.after(10, self.update_textarea) # in 10 milliseconds
def check_password(self, event):
if self.password in event.widget.get():
self.master.destroy() # exit GUI
App(Tk()).master.mainloop()