Maybe this can help you. The below code creates a Main-Frame with a JList
and a JButton
. If you click on the button a Client-Frame will appear and you can modify the names (in JList
).
You just have to work on the same data object (the model)...
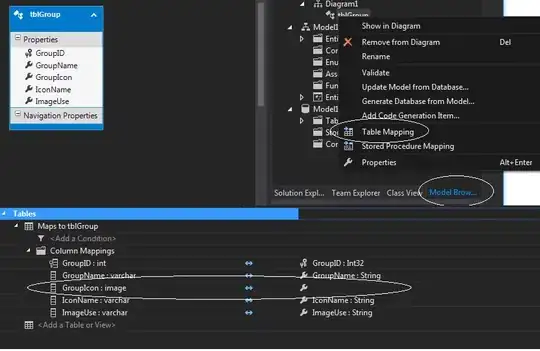
public class TransferDataTest {
public static void main(String[] args) {
new TransferDataTest();
}
public TransferDataTest() {
new MainFrame();
}
}
public class MainFrame extends JFrame {
public MainFrame() {
super("Main");
setLayout(new BorderLayout(4, 4));
// Create a model which you can use for communication between frames
final DefaultListModel<String> model = new DefaultListModel<>();
model.addElement("Mike");
model.addElement("Smith");
JList list = new JList(model);
getContentPane().add(list, BorderLayout.CENTER);
JButton b = new JButton("Change...");
b.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
// Pass over your model so the client will can modify the data
new ClientFrame(model);
}
});
getContentPane().add(b, BorderLayout.SOUTH);
pack();
setVisible(true);
}
}
public class ClientFrame extends JFrame {
public ClientFrame(final DefaultListModel<String> model) {
super("Client");
setLayout(new BorderLayout());
// Init the GUI with data from the model
final JTextField tfFirstName = new JTextField(model.get(0));
final JTextField tfLastiName = new JTextField(model.get(1));
JButton submit = new JButton("Submit");
submit.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
// On submit click update the model with data from the GUI
model.set(0, tfFirstName.getText());
model.set(1, tfLastiName.getText());
}
});
getContentPane().add(tfFirstName, BorderLayout.NORTH);
getContentPane().add(tfLastiName, BorderLayout.CENTER);
getContentPane().add(submit, BorderLayout.SOUTH);
pack();
setLocationRelativeTo(null);
setVisible(true);
}
}