If I get it right (not 100% sure from your drawing) then it is like this:
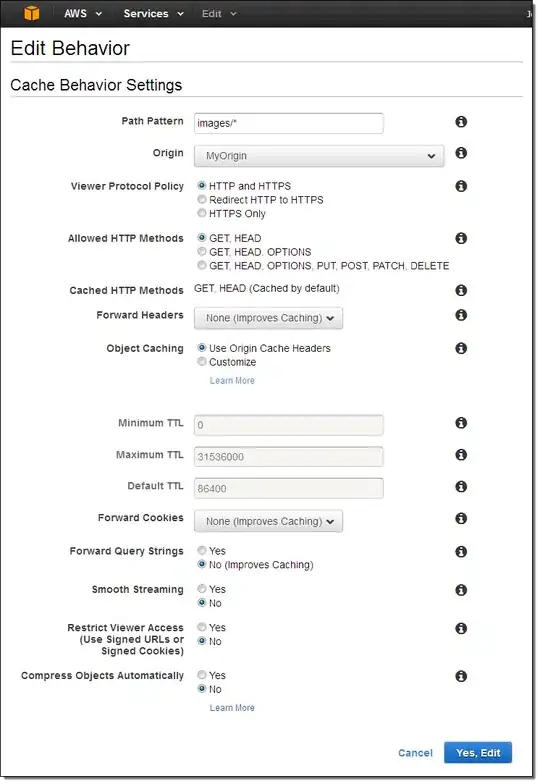
do not test single point
- use actual and last player's position instead
- compute the intersection point
G
between axis given by P0
,P1
- and line
P2
,P3
which is also player movement vector
- if no intersection then no passing
- if none or infinite intersections then player moving along gate not passing (this edge case need to be handled)
one intersection
- this is non edge case of player passing so
- if
|P0-G|<=d
then player is pasing through gate point P0
- if
|P1-G|<=d
then player is pasing through gate point P1
d
is some accuracy distance
- else compute
q=|G-P0|/|P1-P0|
- if 'q<0' then player is passing on left side
- if 'q>|P1-P0|' then player is passing on right side
- if
(q>=0) && (q<=|P1-P0|)
then player is passing in the middle
some C++ code:
double x0=?,x1=?,y0=?,y1=?; // input points (axis)
double x2=?,x3=?,y2=?,y3=?; // input points (line)
double xx0,yy0,xx1,yy1;
double kx0,ky0,dx0,dy0,t0;
double kx1,ky1,dx1,dy1,t1;
kx0=x0; ky0=y0; dx0=x1-x0; dy0=y1-y0;
kx1=x2; ky1=y2; dx1=x3-x2; dy1=y3-y2;
t1=divide(dx0*(ky0-ky1)+dy0*(kx1-kx0),(dx0*dy1)-(dx1*dy0));
xx1=kx1+(dx1*t1);
yy1=ky1+(dy1*t1);
if (fabs(dx0)>=fabs(dy0)) t0=divide(kx1-kx0+(dx1*t1),dx0);
else t0=divide(ky1-ky0+(dy1*t1),dy0);
xx0=kx0+(dx0*t0);
yy0=ky0+(dy0*t0);
// check if intersection exists
if (fabs(xx1-xx0)<=1e-6) // intersection in both lines are the same
if (fabs(yy1-yy0)<=1e-6)
if ((t1<0.0)||(t1>1.0)) // t1 is in <0,1> ... line bounds
{
if (t0<0.0) return "left pass";
if (t0>1.0) return "right pass";
return "middle pass";
}
return "no pass";
divide:
double divide(double x,double y) { if ((y>=-1e-30)&&(y<=+1e-30)) return 0.0; return x/y; }
[notes]
- you do not need normal (I first thought that it would be necessary but it isn't)
- intersection code is extracted from Finding holes in 2d point sets
- so if I forget to copy something there you can find it
- if your player does not go through the axis (instead goes below or above it)
- then you have to modify the intersections and/or project the players points to
P0
,P1
,normal
plane before computations