So...I made a sample project that changes the progress color and you also can change the background progress bar color.
First create a custom progress bar xml file.
custom_progressbar.xml
<!-- Define the background properties like color etc -->
<item android:id="@android:id/background">
<shape>
<gradient
android:startColor="#000001"
android:centerColor="#0b131e"
android:centerY="1.0"
android:endColor="#0d1522"
android:angle="270"
/>
</shape>
</item>
<!-- Define the progress properties like start color, end color etc -->
<item android:id="@android:id/progress">
<clip>
<shape>
<gradient
android:startColor="#2A98E1"
android:centerColor="#2A98E1"
android:centerY="1.0"
android:endColor="#06101d"
android:angle="270"
/>
</shape>
</clip>
</item>
Activity Layout:
activity_my.xml
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
android:paddingBottom="@dimen/activity_vertical_margin"
android:orientation="horizontal"
tools:context=".MyActivity">
<ProgressBar
android:id="@+id/myProgress"
style="?android:attr/progressBarStyleHorizontal"
android:layout_width="match_parent"
android:layout_weight="0.7"
android:layout_height="wrap_content" />
<TextView
android:id="@+id/progressTextView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="None"/>
</LinearLayout>
The test activity:
The important part is this:
Drawable draw=getResources().getDrawable(R.drawable.custom_progressbar);
progressBar.setProgressDrawable(draw);
MyActivity.java
private ProgressBar progressBar;
private Handler handler = new Handler();
private int progressStatus = 0;
private TextView textView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_my);
init();
initProgresBar();
}
public void init(){
textView = (TextView) findViewById(R.id.progressTextView);
progressBar = (ProgressBar) findViewById(R.id.myProgress);
Drawable draw=getResources().getDrawable(R.drawable.custom_progressbar);
// set the drawable as progress drawable
progressBar.setProgressDrawable(draw);
}
public void initProgresBar(){
new Thread(new Runnable() {
public void run() {
while (progressStatus < 100) {
progressStatus += 1;
// Update the progress bar and display the
//current value in the text view
handler.post(new Runnable() {
public void run() {
progressBar.setProgress(progressStatus);
textView.setText(progressStatus+"/"+progressBar.getMax());
}
});
try {
// Sleep for 200 milliseconds.
//Just to display the progress slowly
Thread.sleep(200);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}).start();
}
And the output will be this:
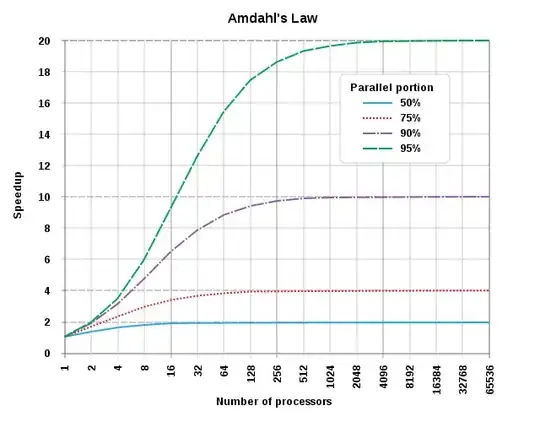
Hope this is what you where looking for. Cheers
For more info you will find this usefull: stack progress bar post