Objects
You have to remember that Rails is object orientated
This means that if you're looking to work with methods, you have to appreciate their relation to the objects you're hoping them to work on. The important thing to note here is that there are therefore two ways you could create the functionality you desire:
- Create a "helper" method
- Create an "instance" method
I'll explain both for you here:
Helper
"Helper" methods are pretty much the same as PHP functions, in that they are ambiguous.
They live in #app/helpers/[x]_helper.rb
, and look like this:
#app/helpers/application_helper.rb
class ApplicationHelper
def my_method
...
end
end
The vital thing to note here is that as helpers are detached from your objects
, you have to send through the data you wish to manipulate. This is very important because, as you'll see in a minute, it means you can use them to process a large variety of different data-sets
Here's how you'd use a helper method in your case:
#View
<%= get_file_name course.name %>
#app/helpers/application_helper.rb
class ApplicationHelper
def get_file_name name
name.squish.downcase.tr(" ","_").gsub(/[^0-9a-z_]/i, '')
end
end
Instance Method
The next step is to look at creating an instance method for your Course
model itself.
Because Rails (by virtue of being built on Ruby) is object orientated, every Model
you create is basically an "object". This means that if you wanted to, you could introduce an instance method for each instance of that object:
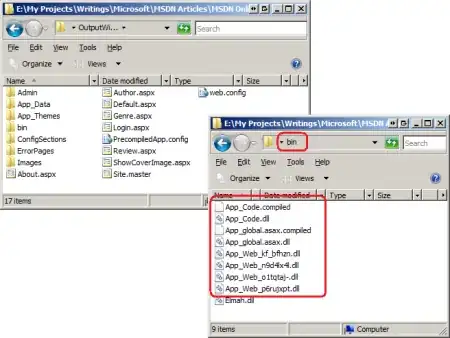
#app/models/course.rb
class Course < ActiveRecord::Base
def get_file_name
name.squish.downcase.tr(" ","_").gsub(/[^0-9a-z_]/i, '')
end
end
This will allow you to call: @course.get_file_name