tl;dr
Use modern java.time classes to parse the inputs into LocalDateTime
objects by defining a formatting pattern with DateTimeFormatter
, and comparing by calling isBefore
.
java.time
The modern approach uses the java.time classes.
Define a formatting pattern to match your inputs.
Parse as LocalDateTime
objects, as your inputs lack an indicator of time zone or offset-from-UTC.
String startInput = "2014/09/12 00:00";
String stopInput = "2014/09/13 00:00";
DateTimeFormatter f = DateTimeFormatter.ofPattern( "uuuu/MM/dd HH:mm" );
LocalDateTime start = LocalDateTime.parse( startInput , f ) ;
LocalDateTime stop = LocalDateTime.parse( stopInput , f ) ;
boolean isBefore = start.isBefore( stop ) ;
Dump to console.
System.out.println( start + " is before " + stop + " = " + isBefore );
See this code run live at IdeOne.com.
2014-09-12T00:00 is before 2014-09-13T00:00 = true
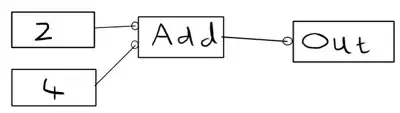
About java.time
The java.time framework is built into Java 8 and later. These classes supplant the troublesome old legacy date-time classes such as java.util.Date
, Calendar
, & SimpleDateFormat
.
To learn more, see the Oracle Tutorial. And search Stack Overflow for many examples and explanations. Specification is JSR 310.
The Joda-Time project, now in maintenance mode, advises migration to the java.time classes.
You may exchange java.time objects directly with your database. Use a JDBC driver compliant with JDBC 4.2 or later. No need for strings, no need for java.sql.*
classes.
Where to obtain the java.time classes?
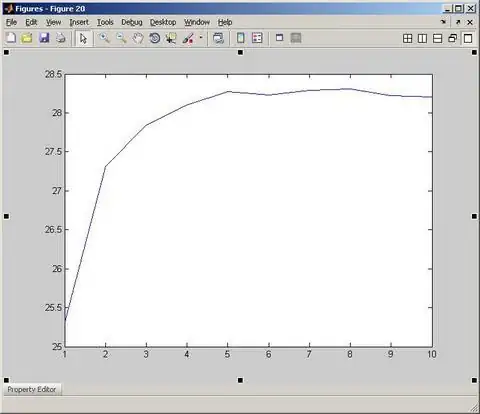
The ThreeTen-Extra project extends java.time with additional classes. This project is a proving ground for possible future additions to java.time. You may find some useful classes here such as Interval
, YearWeek
, YearQuarter
, and more.