Here is a simple example with Swift 3. We have a UITableViewController
in Storyboard set with type grouped and content of type static cells. Its tableView
contains one UITableViewCell
. The cell contains one UILabel
that has a number of lines set to 0 and some very long text to display. The label also has four Auto layout constraints with its superView
(top, bottom, leading and trailing).
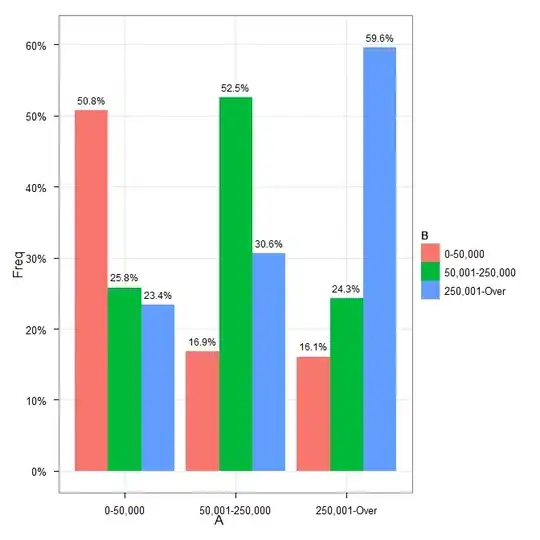
Now, you may choose one of the four following code snippets in order to allow your static cell to automatically resize itself according to its content.
#1. Using tableView(_:estimatedHeightForRowAt:)
and tableView(_:heightForRowAt:)
Note: tableView(_:estimatedHeightForRowAt:)
method requires iOS7
import UIKit
class TableViewController: UITableViewController {
override func tableView(_ tableView: UITableView, heightForRowAt indexPath: IndexPath) -> CGFloat {
return UITableViewAutomaticDimension
}
override func tableView(_ tableView: UITableView, estimatedHeightForRowAt indexPath: IndexPath) -> CGFloat {
return 100 // Return `UITableViewAutomaticDimension` if you have no estimate
}
}
#2. Using estimatedRowHeight
property and tableView(_:heightForRowAt:)
Note: estimatedRowHeight
property requires iOS7
import UIKit
class TableViewController: UITableViewController {
override func viewDidLoad() {
super.viewDidLoad()
tableView.estimatedRowHeight = 100
}
override func tableView(_ tableView: UITableView, heightForRowAt indexPath: IndexPath) -> CGFloat {
return UITableViewAutomaticDimension
}
}
#3. Using tableView(_:heightForRowAt:)
import UIKit
class TableViewController: UITableViewController {
// Bind this IBOutlet to the cell in Storyboard
@IBOutlet weak var cell: UITableViewCell!
override func tableView(_ tableView: UITableView, heightForRowAt indexPath: IndexPath) -> CGFloat {
let fittingSize = CGSize(width: tableView.bounds.size.width, height: 0)
let systemLayoutSize = cell.systemLayoutSizeFitting(fittingSize, withHorizontalFittingPriority: UILayoutPriorityRequired, verticalFittingPriority: UILayoutPriorityFittingSizeLevel)
return systemLayoutSize.height
}
}
#4. Using UITableViewCell
subclass and tableView(_:heightForRowAt:)
import UIKit
class TableViewController: UITableViewController {
// 1. Set custom class to `CustomCell` for cell in Storyboard
// 2. Bind this IBOutlet to the cell in Storyboard
@IBOutlet weak var cell: CustomCell!
override func tableView(_ tableView: UITableView, heightForRowAt indexPath: IndexPath) -> CGFloat {
cell.layoutIfNeeded()
let contentViewSize = cell.contentView.systemLayoutSizeFitting(UILayoutFittingCompressedSize)
return contentViewSize.height + 1 // + 1 for the separator
}
}
class CustomCell: UITableViewCell {
// 1. Set custom class to `CustomCell` for cell in Storyboard
// 2. Bind this IBOutlet to the cell's label in Storyboard
@IBOutlet weak var label: UILabel!
override func layoutSubviews() {
super.layoutSubviews()
label.preferredMaxLayoutWidth = frame.width
}
}
All four previous code snippets will result in the following display:
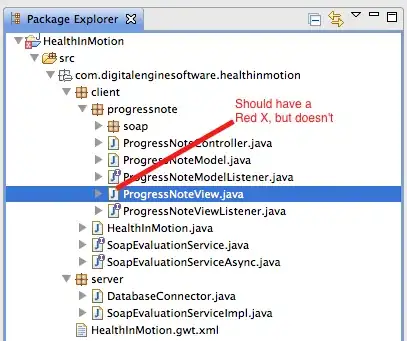