I have done this before by using a stored procedure for building my query, which will allow you have lots of order_id columns. I assume you are using MySQL.
This shows how to output the order_id
, you could do the same for output the brand
too.
The sqlfiddle
create table events and data
create table Customer (customer_id int);
create table Orders (customer_id int, order_id int, brand char, order_date date);
insert into customer values(1);
insert into customer values(2);
insert into customer values(3);
insert into orders values(1, 101,'A', '2010-02-01');
insert into orders values(1, 102,'B', '2010-02-05');
insert into orders values(1, 103,'A', '2014-01-06');
insert into orders values(2, 204,'B', '2013-01-02');
insert into orders values(2, 205,'D', '2013-02-02');
insert into orders values(3, 306,'A', '2013-03-07');
insert into orders values(3, 307,'B', '2013-04-07');
insert into orders values(3, 308,'C', '2013-05-07');
insert into orders values(3, 309,'D', '2013-07-07');
insert into orders values(3, 310,'E', '2013-11-07');
insert into orders values(3, 311,'F', '2013-11-07');
create function buildQuery()
create function buildQuery() returns varchar(4000)
not deterministic
reads sql data
begin
-- variables
declare query varchar(4000);
declare maxcols int;
declare counter int;
-- initialize
set query = '';
set maxcols = 0;
set counter = 0;
-- get the max amount of columns
select count(distinct order_id) as maxorders into maxcols
from Orders
group by customer_id
order by maxorders desc limit 1;
-- build the query
while counter < maxcols do
set counter = counter + 1;
set query=concat(query,',replace(substring(substring_index(group_concat(order_id), '','',', counter,'),length(substring_index(group_concat(order_id),'','',', counter,'-1)) + 1),'','','''') as order' ,counter);
end while;
-- return
return query;
end//
execute the function
set @q = buildQuery();
set @q = concat('select customer_id ', @q, '
from Orders
group by customer_id');
prepare s from @q;
execute s;
deallocate prepare s;
run results
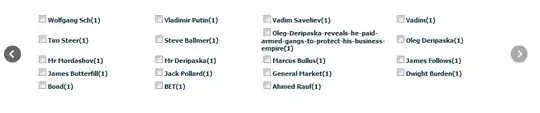
SqlFiddle
The sqlfiddle