Something like this might help,
dat <- data.frame(x = 1:10, y = 1:10)
p <- ggplot(dat, aes(x=x, y=y)) + geom_point() +
scale_x_continuous('', breaks=NULL)+
scale_y_continuous('', breaks=NULL)
g <- ggplotGrob(p)
library(gtable)
library(grid)
my_axis <- function(low="low", high="high", axis=c("x", "y"), ...){
axis <- match.arg(axis)
if(axis == "x"){
g1 <- textGrob(low, x=unit(0,"npc"), hjust=0)
g3 <- textGrob(high, x=unit(1,"npc"), hjust=1)
g2 <- segmentsGrob(grobWidth(g1) + unit(2,"mm"), unit(0.5,"npc"),
unit(1,"npc") - grobWidth(g3)- unit(2,"mm"),
unit(0.5,"npc"), ...)
} else if(axis == "y"){
g1 <- textGrob(low, y=unit(0,"npc"), rot=90, hjust=0)
g3 <- textGrob(high, y=unit(1,"npc"), rot=90, hjust=1)
g2 <- segmentsGrob(unit(0.5,"npc"),grobHeight(g1) + unit(2,"mm"),
unit(0.5,"npc"),
unit(1,"npc") - grobHeight(g3)- unit(2,"mm"),
...)
}
grobTree(g1,g2,g3)
}
g <- gtable_add_grob(g, my_axis(arrow=arrow(length=unit(2,"mm"))),
t=nrow(g)-2, b=nrow(g)-1, l=4)
g <- gtable_add_grob(g, my_axis(axis="y", arrow=arrow(length=unit(2,"mm"))),
t=3, l=1,r=3)
grid.newpage()
grid.draw(g)
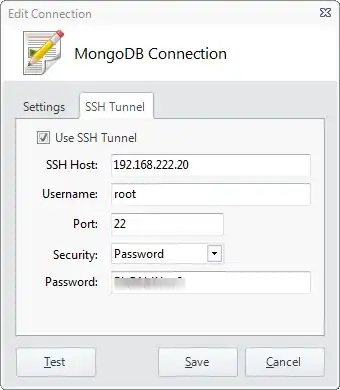