first off, I would consider LINQ slow. LINQ is pretty much a clean way to nest array iteration logic. Only use when the CPU must manipulate static data. or data is CPU generated and there is no backing storage.
that's how I think of it anyways. now for the answer:
I have built a database in SQLServer 2012 Express for Demon-Striation purposes (<- Xanth reference). I used your browsers and made imaginary users. the pivot code should be a stored procedure you call from c#. if you are using VS I can edit for a pure VS(2012) solution because I would prefer to use datasets and add a query to the TableAdapter for using a stored procedure. but this should get you 2/3rds the way there
the User Table::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::
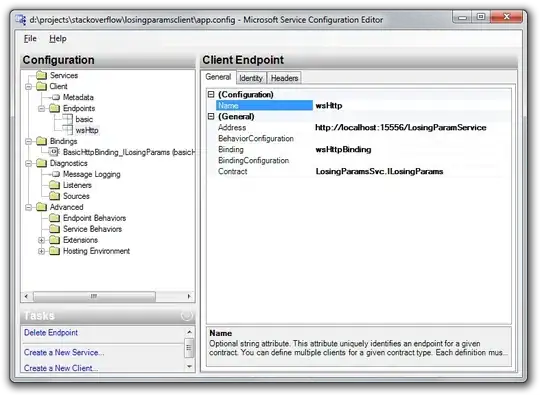
the Browser Table:::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::
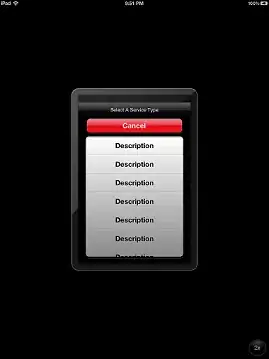
the Usage Table Sample (324 row total):::::::::::::::::::::::::::::::::::::::::::::::::::
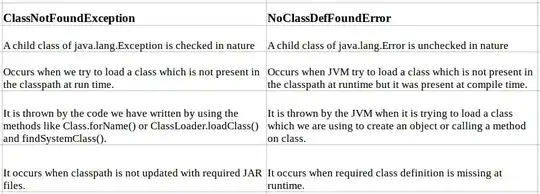
the Diagram:::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::
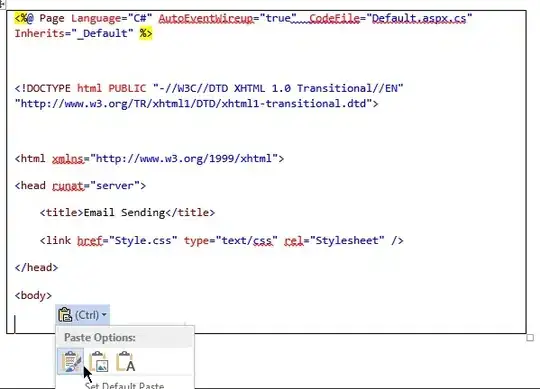
The PIVOT (I added this as a stored procedure):::::::::::::::::::::::::::::::::::::::::::
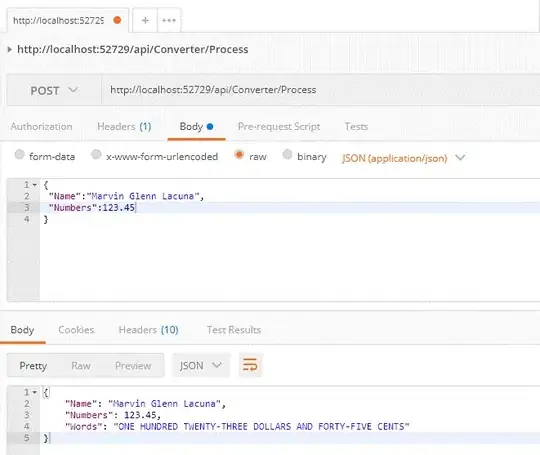
VS 2012 / WPF implementation::::::::::::::::::
after adding the stored procedure, connecting VS to your database, and adding a Dataset to your project. Drag and drop the stored procedure into your dataset. you can also use the stored procedure without the typed dataset generator. See Here

WPF XAML::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::
<Window x:Class="WPFPIVOT.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="MainWindow" Height="350" Width="525">
<Grid>
<DataGrid Name="datagrid" ItemsSource="{Binding}"/>
</Grid>
</Window>
.cs::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Data;
namespace WPFPIVOT
{
/// <summary>
/// Interaction logic for MainWindow.xaml
/// </summary>
public partial class MainWindow : Window
{
Pivot pivoted;
PivotTableAdapters.GetUsageTableAdapter adapter;
public MainWindow()
{
InitializeComponent();
//this code is the same for WPF and FORMs
pivoted = new Pivot();
adapter = new PivotTableAdapters.GetUsageTableAdapter();
adapter.Fill(pivoted.GetUsage);
///////////////////////////////////////////////////////////////
datagrid.DataContext = pivoted;
}
}
}
WPF WINDOW:::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::::
(the UserID column defaults to the right most column the select statement could be select p1.UserID,p1[1],... p.[n]
)
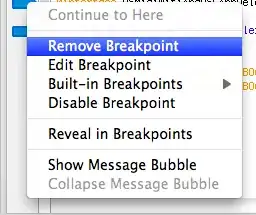