keyCode
should be readonly in all modern browsers, simply because modifying it is hacky and unnecessary. Looking at the code you provided in your other question: keydown event does not fire correctly using jQuery trigger, we can imitate this functionality rather than attempt to return the altered event.
jQuery actually does let us modify the event
object when it is passed in to a jQuery event handler. As you have jquery tagged, we can therefore make use of this in our answer, passing the modifications into a trigger()
called on the same element.
if (e.which === 13) {
// Alter the normalised e.which property
e.which = 9;
// Alter the default properties for good measure
e.charCode = 9;
e.keyCode = 9;
$(this).trigger(e);
return false;
}
Note: I've used e.which
rather than e.keyCode
as jQuery normalises this across all browsers.
Here is a demo which shows this in action. If you press the Enter key when the input
element is in focus, the event will be replaced with the Tab event:
var $input = $('input'),
$p = $('p');
$input.on('keydown', function(e) {
if (e.which == 13) {
$p.html($p.html() + 'Enter key intercepted, modifying 13 to 9.<br>');
e.which = 9;
e.charCode = 9;
e.keyCode = 9;
$(this).trigger(e);
return false;
}
$p.html($p.html() + 'Key pressed: ' + e.keyCode + '<br>');
});
<script src="https://ajax.googleapis.com/ajax/libs/jquery/1.11.1/jquery.min.js"></script>
<input type="text" />
<p></p>
As I've mentioned in my answer here, just because you're changing the event doesn't mean your browser is then going to handle it as if the Tab key has been pressed. The logging lets us know that our event has successfully been intercepted and changed from Enter to Tab, even on Internet Explorer 11.
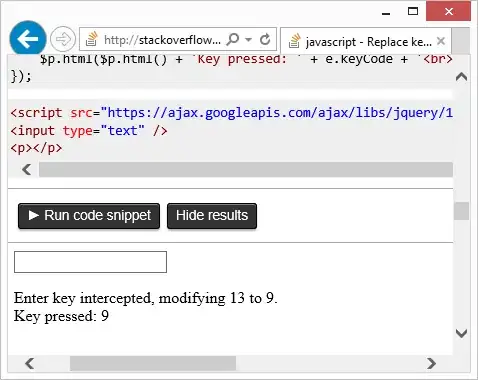