AUTO_INCREMENT is a special value which may differ from the ID of the last record.
- If records deleted, the AUTO_INCREMENT will still continue.
- If records failed to insert, the AUTO_INCREMENT will still continue.
Assume you are using MySQL, you can see its value in phpMyAdmin.
- Open a database, then a table.
- Switch to the Operations tab.

- Find the AUTO_INCREMENT field under Table options.
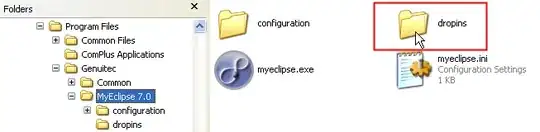
So you should obtain its value, especially when you depend on the AUTO_INCREMENT of your database.
For inserting one record, getting the LAST_INSERT_ID()
could be an option. But I still don't prefer to do triple operations (insert, read ID, update). Better to minimize the I/O operations (read AUTO_INCREMENT, insert).
In my case, I need to import data from an Excel document. Surely, when performance is important, I cannot depend on the ActiveRecord model. So I use batchInsert() command.
I am adopting this answer for getting the AUTO_INCREMENT value in MySQL.
$lastModelId = Yii::$app->db->createCommand("
SELECT `AUTO_INCREMENT`
FROM INFORMATION_SCHEMA.TABLES
WHERE TABLE_SCHEMA = DATABASE()
AND TABLE_NAME = 'my_table'
")->queryScalar();
If using table prefix:
$lastModelId = Yii::$app->db->createCommand("
SELECT `AUTO_INCREMENT`
FROM INFORMATION_SCHEMA.TABLES
WHERE TABLE_SCHEMA = DATABASE()
AND TABLE_NAME = :TableName
")->bindValues([
':TableName' => MyModel::getTableSchema()->name,
])->queryScalar();