Be aware! float
and double
can break your heart when used with a calculator. These types are not for doing taxes or balancing a checkbook. Why? Because they don't use 10 digit numbers like you and I. They use binary numbers. With whole numbers that's no problem but with fractions you run into problems that are hard to see because java ACCEPT'S them in base 10, DISPLAY'S them in base 10, but it doesn't STORE them in base 10.
That can make it hard to realize that there is no such thing as 0.1 in a float
or double
. It's as impossible to perfectly express as π is for us in base 10. When you pull it back out it might display that way because of rounding but that is not what was stored and that is not what you'll be calculating against. This is why Calculators do NOT use the IEEE floats and doubles. These types are intended for use with scientific instruments for taking measurements and doing calculations that are fine with precision limits. If you are modeling a calculator like you used in grade school take note that those do NOT use IEEE floats or doubles for this very reason.
For more about this issue read this and this
Instead some very smart people have created something that's perfect for modeling calculators: java.math.BigDecimal;
If you're the visual type let me show you something that might make the difference between BigDecimal and float and double clear:
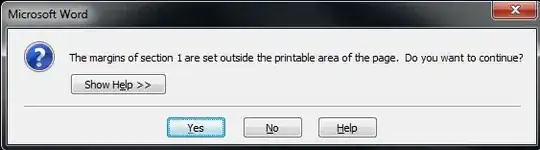
These rulers both show inches. For whole inches there is no difference between them. However, they divide inches differently. The top one divides by 10's. The bottom one divides by 2's. The top can be perfectly modeled by BigDecimal. The bottom can be perfectly modeled by float or double. Some of the ticks will line up like .5 because 5/10 = 1/2 but 1/10 = x / (2^n) doesn't have a solution where x and n are whole numbers.
Because humans have a long tradition of using base ten numbers in calculators I don't recommend letting floats or doubles anywhere near your accountant.
import java.math.BigDecimal;
import java.util.Scanner;
public class javaProject
{
public static void main(String[] args)
{
BigDecimal x;
BigDecimal y;
String whatOp;
Scanner input = new Scanner(System.in);
System.out.println("please enter the first number");
x = input.nextBigDecimal();
System.out.println("please enter the second number");
y = input.nextBigDecimal();
Scanner op = new Scanner(System.in);
System.out.println("Please enter the operation you want to use");
whatOp = op.next();
if (whatOp.equals("+"))
{
System.out.println( "The answer is " + ( x.add(y) ) );
}
else if (whatOp.equals("-"))
{
System.out.println( "The answer is " + ( x.subtract(y) ) );
}
else if (whatOp.equals("*"))
{
System.out.println( "The answer is " + ( x.multiply(y) ) );
}
else if (whatOp.equals("/"))
{
System.out.println( "The answer is " + ( x.divide(y) ) );
}
else
{
System.out.println("Thats not a choice!");
}
}
}