actually you don't need a multiple frame for this.you don't need even multiple jpanels .just a single jlable is sufficient .
all you need to do is change image of jlable when button press .
1) put your images in a directory and give a name .names should have pattern
example
C:\Users\Madhawa.se\Desktop\images\i1.jpg
C:\Users\Madhawa.se\Desktop\images\i2.jpg
C:\Users\Madhawa.se\Desktop\images\i3.jpg
C:\Users\Madhawa.se\Desktop\images\i4.jpg
C:\Users\Madhawa.se\Desktop\images\i5.jpg
2) declare a int variable as global
int i=0;
3) increase i by one when click next button and add appropriate image to label
i++;
ImageIcon imgThisImg = new ImageIcon("C:\\Users\\Madhawa.se\\Desktop\\images\\i"+i+".jpg");
jLabel1.setIcon(imgThisImg);
4) decrease i by one when click previous button and add appropriate image to label
i--;
ImageIcon imgThisImg = new ImageIcon("C:\\Users\\Madhawa.se\\Desktop\\images\\i"+i+".jpg");
jLabel1.setIcon(imgThisImg);
you can declare also String array which holds image paths .if your image names want to be arbitrary names .and then you can get image same way.
and also you should have a logic to correctly cycle images.you should restrict i to image quantity .otherwise image doesn't exist for i after i exceeded
output>>
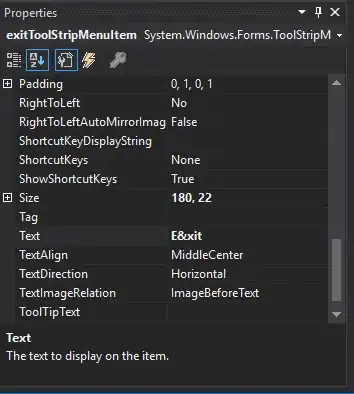