Highlighter#addHighlight
returns an Object
tag which represents the current highlight. This tag should be used when calling Highlighter#removeHighlight
, this, I assume, means you can use the same instance of the HighlightPainter
to highlight multiple parts of the document, but still manage them separately, for example...
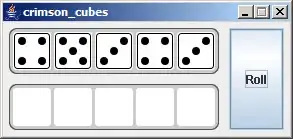
import java.awt.BorderLayout;
import java.awt.Color;
import java.awt.EventQueue;
import java.awt.GridBagConstraints;
import java.awt.GridBagLayout;
import java.awt.Rectangle;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.io.File;
import java.io.FileReader;
import java.io.IOException;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.JScrollPane;
import javax.swing.JTextField;
import javax.swing.JTextPane;
import javax.swing.UIManager;
import javax.swing.UnsupportedLookAndFeelException;
import javax.swing.text.BadLocationException;
import javax.swing.text.DefaultHighlighter;
import javax.swing.text.Document;
public class TestEditorPane01 {
public static void main(String[] args) {
new TestEditorPane01();
}
public TestEditorPane01() {
EventQueue.invokeLater(new Runnable() {
@Override
public void run() {
try {
UIManager.setLookAndFeel(UIManager.getSystemLookAndFeelClassName());
} catch (ClassNotFoundException | InstantiationException | IllegalAccessException | UnsupportedLookAndFeelException ex) {
}
JFrame frame = new JFrame();
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setLayout(new BorderLayout());
frame.add(new EditorPane());
frame.setSize(400, 400);
frame.setLocationRelativeTo(null);
frame.setVisible(true);
}
});
}
public class EditorPane extends JPanel {
private JTextPane editor = new JTextPane();
private int lastMatch;
private String find = "Method";
private DefaultHighlighter.DefaultHighlightPainter highlightPainter;
private Object highlightTag;
private JTextField searchField;
private JButton searchButton;
public EditorPane() {
setLayout(new BorderLayout());
editor = new JTextPane();
try (FileReader reader = new FileReader(new File("/some file.txt"))) {
editor.read(reader, "script");
} catch (IOException exp) {
exp.printStackTrace();
}
add(new JScrollPane(editor));
JPanel searchPane = new JPanel(new GridBagLayout());
searchField = new JTextField(10);
searchButton = new JButton("Search");
GridBagConstraints gbc = new GridBagConstraints();
gbc.gridx = 0;
gbc.gridy = 0;
gbc.fill = GridBagConstraints.HORIZONTAL;
gbc.weightx = 1;
searchPane.add(searchField, gbc);
gbc.gridx++;
gbc.fill = GridBagConstraints.NONE;
gbc.weightx = 0;
searchPane.add(searchButton, gbc);
searchButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
String text = searchField.getText();
if (!text.equals(find)) {
find = text;
lastMatch = 0;
}
highlightNext();
}
});
add(searchPane, BorderLayout.SOUTH);
}
public void highlightNext() {
Document document = editor.getDocument();
try {
if (lastMatch + find.length() >= document.getLength()) {
lastMatch = 0;
}
for (; lastMatch + find.length() < document.getLength(); lastMatch++) {
String match = document.getText(lastMatch, find.length());
if (find.equalsIgnoreCase(match)) {
if (highlightTag != null) {
editor.getHighlighter().removeHighlight(highlightTag);
}
if (highlightPainter == null) {
highlightPainter = new javax.swing.text.DefaultHighlighter.DefaultHighlightPainter(Color.YELLOW);
}
highlightTag = editor.getHighlighter().addHighlight(lastMatch, lastMatch + find.length(), highlightPainter);
Rectangle viewRect = editor.modelToView(lastMatch);
editor.scrollRectToVisible(viewRect);
lastMatch += find.length();
break;
}
}
} catch (BadLocationException ex) {
ex.printStackTrace();
}
}
}
}
Since you are doing this, and it's not working, this would suggest that there is something else wrong with your code which is not evident in the snippet you have provided. Consider providing a runnable example which demonstrates your problem. This will result in less confusion and better responses
Updated...
- Don't link your example code to an external site, not everyone can access external sites or can be bothered to follow links anyway...
- A runnable example should be self contained and have no reliance on other libraries or resources, like icons and should be contained within a single file
- Don't expose your UI components unnecessarily, that is, I don't think
SearchDialog
really needs to know about TestFrame
, all it's interested in is the JTextComponent
...
- Your "new" example and your existing code snippet are inconsistent. You are no longer assigning the result of
addHighlight
to last
??
- Don't use
null
layouts...
When I finally got your example code to compile, this is what I was presented with...
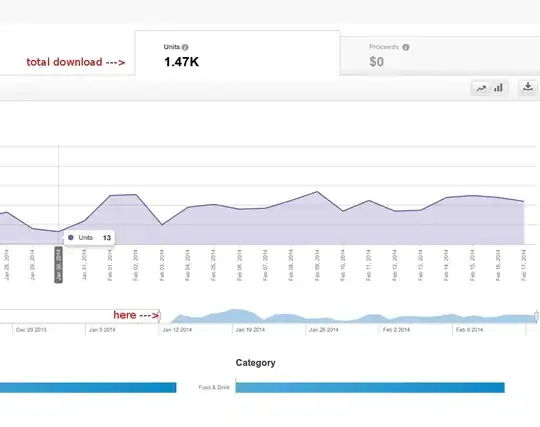
...Okay, so thought, I'd just expand the window...
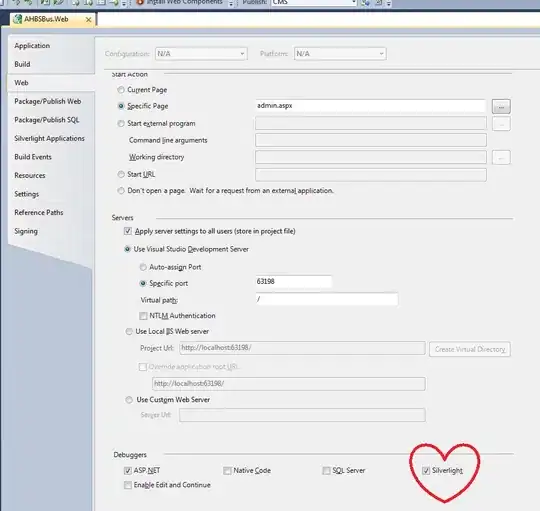
...well, there's a problem...which I don't have the time to solve.
Avoid using null
layouts, pixel perfect layouts are an illusion within modern ui design. There are too many factors which affect the individual size of components, none of which you can control. Swing was designed to work with layout managers at the core, discarding these will lead to no end of issues and problems that you will spend more and more time trying to rectify
Take a look at Why is it frowned upon to use a null layout in SWING? for more details...