You have multiple errors in your program. For one, the variable prt
should probably be ptr
. Also, your while condition should be using the &&
boolean operator instead of the &
bitwise operator. From your description, it appears that the last two lines of your loop:
NewNode -> next = ptr -> next;
ptr -> next = NewNode;
Should only be invoked if count == 3
. However, in it's current form they are being executed on every loop iteration. Likewise, you should only create a NewNode
when you actually plan to do the insertion.
You're also checking the condition count == 3
multiple times including indirectly through the found
variable. Each of these conditions could be collapsed into a single if
like so:
if (count == 3) {
found = true;
Node NewNode = new Node;
NewNode->info = 10;
NewNode->next = ptr->next;
ptr->next = NewNode;
} else {
ptr = ptr->next;
}
You should strongly think about any loop that you write and what loop invariants you want to maintain through the loop as well as what thing is going to change on every loop iteration so that you can have a deterministic end to your loop.
Also, whenever you are dealing with a new type of data structure that you have never worked with before, I recommend drawing out a little diagram with boxes and arrows. This will help you figure out the process which you need to follow to get what you want.
I would start with an initial diagram and then a final diagram showing the picture you want. Then draw a marker that corresponds to the pointer that iterates across your list. Move the marker one time through the loop on each step. Then try to implement the steps you took with the marker in code form.
Here's some example starting diagrams that I drew using Google Docs:
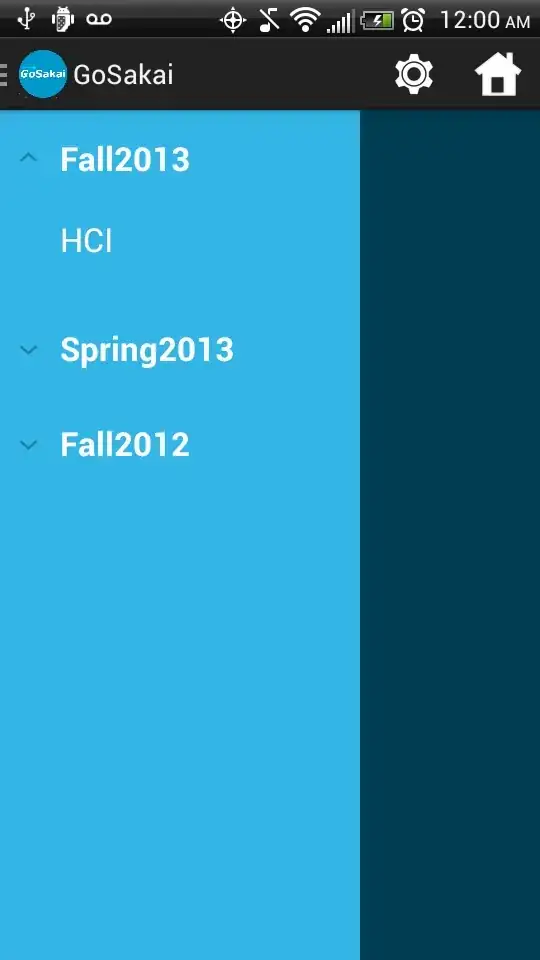
Assume the dotted arrows represent NULL pointers. This gives you three potential starting cases for your linked list.