You could physically modify the look and feel's font settings...
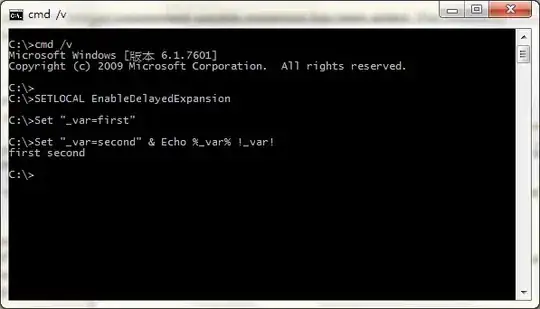
import java.awt.EventQueue;
import java.awt.Font;
import java.util.Set;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.UIManager;
import javax.swing.UnsupportedLookAndFeelException;
public class Test {
public static void main(String[] args) {
new Test();
}
public Test() {
EventQueue.invokeLater(new Runnable() {
@Override
public void run() {
try {
UIManager.setLookAndFeel(UIManager.getSystemLookAndFeelClassName());
} catch (ClassNotFoundException | InstantiationException | IllegalAccessException | UnsupportedLookAndFeelException ex) {
ex.printStackTrace();
}
setDefaultSize(24);
JFrame frame = new JFrame("Testing");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.add(new JLabel("Happy as a pig in a blanket"));
frame.pack();
frame.setLocationRelativeTo(null);
frame.setVisible(true);
}
});
}
public static void setDefaultSize(int size) {
Set<Object> keySet = UIManager.getLookAndFeelDefaults().keySet();
Object[] keys = keySet.toArray(new Object[keySet.size()]);
for (Object key : keys) {
if (key != null && key.toString().toLowerCase().contains("font")) {
System.out.println(key);
Font font = UIManager.getDefaults().getFont(key);
if (font != null) {
font = font.deriveFont((float)size);
UIManager.put(key, font);
}
}
}
}
}
We use a similar approach to increase/decrease the font size of the running application to test layouts (and eventually allow the user some additional control over the font size)