tl;dr
I want to get current day i.e 12, current month i.e November, current year i.e 2014 and hour, minute, not seconds and AM or PM.
ZonedDateTime
.now(
ZoneId.of( "Pacific/Auckland" ) // Or "Asia/India", "Africa/Tunis", etc.
)
.format(
DateTimeFormatter
.ofPattern(
"dd MMMM uuuu HH:mm"
)
.withLocale(
Locale.CANADA_FRENCH // Or `Locale.US`, or `new Locale( "en" , "IN" )` for English language with India culture.
)
)
See this code run live at IdeOne.com.
28 septembre 2019 08:48
java.time
From these 3 questions I have learnt that to get date and time, we need to use Calendar.
Nope.
The Calendar
class is terrible, along with Date
& SimpleDateFormat
and such. These classes were all supplanted years ago by the modern java.time classes defined in JSR 310.
Now my question is I want to get current day i.e 12, current month i.e November, current year i.e 2014 and hour, minute, not seconds and AM or PM.
Determining a date and time-of-day requires a time zone. For any given moment, the date varies around the globe by zone. For example, a few minutes after midnight in Paris France is a new day while still “yesterday” in Montréal Québec.
If no time zone is specified, the JVM implicitly applies its current default time zone. That default may change at any moment during runtime(!), so your results may vary. Better to specify your desired/expected time zone explicitly as an argument. If you want to depend on the JVM’s current default time zone, make your intention clear by calling ZoneId.systemDefault()
. If critical, confirm the zone with your user.
Specify a proper time zone name in the format of Continent/Region
, such as America/Montreal
, Africa/Casablanca
, or Pacific/Auckland
. Never use the 2-4 letter abbreviation such as EST
or IST
as they are not true time zones, not standardized, and not even unique(!).
ZoneId z = ZoneId.of( "Asia/Kolkata" ) ;
Capture the current moment as seen in the wall-clock time used by the people of a certain region (a time zone).
ZonedDateTime zdt = ZonedDateTime.now( z ) ;
If you do not care about the seconds or fractional-second, truncate.
ZonedDateTime zdt = ZonedDateTime.now( z ).truncatedTo( ChronoUnit.MINUTES ) ;
Generate a string in standard ISO 8601 format, wisely extended to append the name of the time zone in square brackets.
String output = zdt.toString() ;
2019-09-28T01:42+05:30[Asia/Kolkata]
Generate a string, automatically localized.
Locale locale = new Locale( "en" , "IN" ) ; // English language, India culture.
DateTimeFormatter f = DateTimeFormatter.ofLocalizedDateTime( FormatStyle.LONG ).withLocale( locale ) ;
String output2 = zdt.format( f ) ;
28 September 2019 at 1:42:00 AM IST
See this code run live at IdeOne.com.
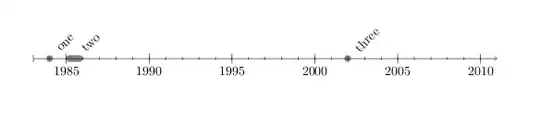
About java.time
The java.time framework is built into Java 8 and later. These classes supplant the troublesome old legacy date-time classes such as java.util.Date
, Calendar
, & SimpleDateFormat
.
To learn more, see the Oracle Tutorial. And search Stack Overflow for many examples and explanations. Specification is JSR 310.
The Joda-Time project, now in maintenance mode, advises migration to the java.time classes.
You may exchange java.time objects directly with your database. Use a JDBC driver compliant with JDBC 4.2 or later. No need for strings, no need for java.sql.*
classes.
Where to obtain the java.time classes?
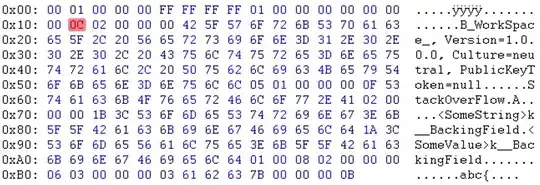