Another possibility could be to put your code to ClassModule and to use Events to callback to WaitMessage user form. Here short example. HTH
Standard module creates the form and the updater object and displays the form which starts processing:
Public Sub Main()
Dim myUpdater As Updater
Dim myRange As Range
Dim myWaitMessage As WaitMessage
Set myRange = ActiveSheet.UsedRange.Cells
Set myUpdater = New Updater
Set myUpdater.SourceRange = myRange
' create and initialize the form
Set myWaitMessage = New WaitMessage
With myWaitMessage
.Caption = "Wait message"
Set .UpdaterObject = myUpdater
' ... etc.
.Show
End With
MsgBox "Module2.Label_ProcessComplete"
End Sub
Class module containes the monitored method and has events which are raised if progress updated or finished. In the event some information is send to the form, here it is the number of processed cells but it can be anything else:
Public Event Updated(updatedCellsCount As Long)
Public Event Finished()
Public CancelProcess As Boolean
Public SourceRange As Range
Public Sub UpdateSheetButton()
Dim subStr1 As String
Dim subSrrt2() As String
Dim tmp As Integer
Dim pos As Integer
Dim changesCount As Long
Dim myCell As Range
Dim Status
' process task and call back to form via event and update it
For Each myCell In SourceRange.Cells
' check CancelProcess variable which is set by the form cancel-process button
If CancelProcess Then _
Exit For
subStr1 = "" ' RemoveTextBetween(Cell.Formula, "'C:\", "\AddIns\XL-EZ Addin.xla'!")
tmp = Len(subStr1) < 1
If tmp >= 0 Then
myCell.Formula = subStr1
Status = True
End If
changesCount = changesCount + 1
RaiseEvent Updated(changesCount)
DoEvents
Next
RaiseEvent Finished
End Sub
User form has instance of updater class declared with 'WithEvent' keyword and handles events of it. Here form updates a label on 'Updated' event and unloads itself on 'Finished' event:
Public WithEvents UpdaterObject As Updater
Private Sub UpdaterObject_Finished()
Unload Me
End Sub
Private Sub UpdaterObject_Updated(updatedCellsCount As Long)
progressLabel.Caption = updatedCellsCount
End Sub
Private Sub UserForm_Activate()
UpdaterObject.UpdateSheetButton
End Sub
Private Sub cancelButton_Click()
UpdaterObject.CancelProcess = True
End Sub
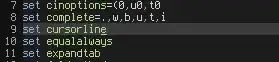