It's not the best practice, but if you really want that functionality, you can just disallow the touch event of the parent scrollview once you touch it and re allow it once you leave the child container.
This is how I have done it before, where "listScanners" is my listview:
listScanners.setOnTouchListener(new View.OnTouchListener()
{
@Override
public boolean onTouch(View v, MotionEvent event)
{
v.getParent().requestDisallowInterceptTouchEvent(true);
return false;
}
});
And this is the part of my layout that is relevant to the question:
/* Theres more before this ... */
<ScrollView
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:scrollbarSize="0dp" >
<LinearLayout
android:orientation="vertical"
android:layout_width="fill_parent"
android:layout_height="fill_parent">
<RelativeLayout
android:orientation="vertical"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:layout_marginTop="5dp"
android:layout_gravity="center"
android:gravity="center"
android:background="@null"
>
<TextView
android:id="@+id/emptylist1"
android:layout_width="450dp"
android:layout_height="80dp"
android:textColor="#6f6f6f"
android:textSize="20sp"
android:padding="3dp"
android:layout_marginBottom="3dp"
android:layout_marginLeft="10dp"
android:layout_marginRight="10dp"
android:text="No Scanners have been added..."/>
<ListView
android:id="@+id/listview_scanners"
android:layout_width="450dp"
android:layout_height="80dp"
android:padding="3dp"
android:layout_marginBottom="10dp"
android:layout_marginLeft="10dp"
android:layout_marginRight="10dp"
android:cacheColorHint="#00000000"
android:divider="#DEDEDE"
android:dividerHeight="1px">
</ListView>
</RelativeLayout>
/* Theres more after this ... */
Correct Way:
Ideally you should rather have something like this, where your layout looks like this:
Where it is very important to have this in your linear layout:
android:isScrollContainer="true"
isScrollContainer means that the linearlayout contains a view that scrolls, meaning that it can be a certain size within the linear layout, however, it may contain much more when you scroll it. Here is the layout:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:background="#afafaf"
android:isScrollContainer="true">
<LinearLayout
android:orientation="horizontal"
android:layout_width="fill_parent"
android:background="#FF63FF9B"
android:layout_height="50dp"
>
<TextView
android:id="@+id/textview_heading"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textSize="25sp"
android:layout_marginTop="5dp"
android:layout_marginLeft="10dp"
android:textColor="#ffffff"
android:text="View First List"/>
</LinearLayout>
<RelativeLayout
android:orientation="vertical"
android:layout_width="fill_parent"
android:layout_height="150dp"
android:layout_marginTop="5dp"
android:background="@null"
>
<ListView
android:id="@+id/listview1"
android:layout_width="fill_parent"
android:layout_height="130dp"
android:cacheColorHint="#00000000"
android:divider="#DEDEDE"
android:dividerHeight="1px">
</ListView>
</RelativeLayout>
<LinearLayout
android:orientation="horizontal"
android:layout_width="fill_parent"
android:background="#FF63FF9B"
android:layout_height="50dp"
>
<TextView
android:id="@+id/textview_heading"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textSize="25sp"
android:layout_marginTop="5dp"
android:layout_marginLeft="10dp"
android:textColor="#ffffff"
android:text="View Second List"/>
</LinearLayout>
<RelativeLayout
android:orientation="vertical"
android:layout_width="fill_parent"
android:layout_height="150dp"
android:layout_marginTop="5dp"
android:background="@null"
>
<ListView
android:id="@+id/listview2"
android:layout_width="fill_parent"
android:layout_height="130dp"
android:cacheColorHint="#00000000"
android:divider="#DEDEDE"
android:dividerHeight="1px">
</ListView>
</RelativeLayout>
<LinearLayout
android:orientation="horizontal"
android:layout_width="fill_parent"
android:background="#FF63FF9B"
android:layout_height="50dp"
>
<TextView
android:id="@+id/textview_heading"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textSize="25sp"
android:layout_marginTop="5dp"
android:layout_marginLeft="10dp"
android:textColor="#ffffff"
android:text="View Third List"/>
</LinearLayout>
<RelativeLayout
android:orientation="vertical"
android:layout_width="fill_parent"
android:layout_height="150dp"
android:layout_marginTop="5dp"
android:background="@null"
>
<ListView
android:id="@+id/listview3"
android:layout_width="fill_parent"
android:layout_height="130dp"
android:cacheColorHint="#00000000"
android:divider="#DEDEDE"
android:dividerHeight="1px">
</ListView>
</RelativeLayout>
</LinearLayout>
I just made this in 5 minutes and you get a layout looking like this: (this is just a draft, obviously just to give you an idea)
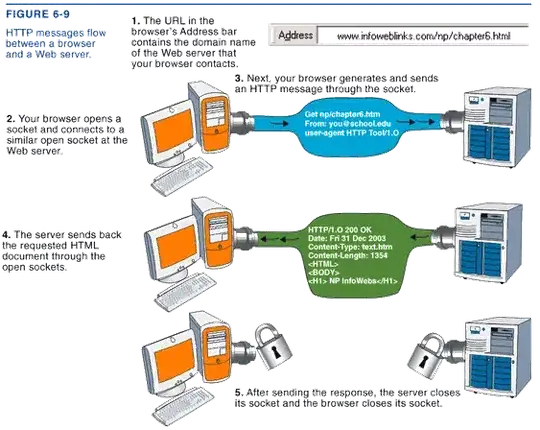
And last but not least, in your code where you display the lists, you will have something like this:
public class Screen_View_Lists extends Activity
{
BaseAdapter listAdapter;
ListView list1,list2,list3;
@Override
public void onCreate(Bundle icicle)
{
super.onCreate(icicle);
setContentView(R.layout.screen_view_packages);
list1 = (ListView) findViewById(R.id.listview1);
list2 = (ListView) findViewById(R.id.listview2);
list2 = (ListView) findViewById(R.id.listview3);
listAdapter = new Adapter_List_Main(this, packages);//This is my own adapter, you probably use your own custom one as well.
list1.setAdapter(listAdapter);
//Setup list to support context menu
registerForContextMenu(list1);
//Setup list to support long click events.
list1.setLongClickable(true);
//Action Listener for long click on item in the list
list1.setOnItemLongClickListener(new AdapterView.OnItemLongClickListener()
{
public boolean onItemLongClick(AdapterView<?> parent, View v, int position, long id)
{
//do things here
}
});
//Action Listener for short click on item in the list
list1.setOnItemClickListener(new AdapterView.OnItemClickListener()
{
public void onItemClick(AdapterView<?> parent, View v, int position, long id)
{
//do things here
}
});
//And so one . . .
}
}