This public string Name { get; set; }
is called an auto implemented property. The compiler will create a private backing field that will hold the value we will set using this property. Furthermore, it will create two methods, one for the set
(setting the value) and one for the get
(getting the value) of this value. You could consider that set
is an assignment and get
is a read. So when we say that we set
a value actually we mean we assign a value to a variable. While when we say that we get
a value actually we mean that we read the value that is stored in a variable.
Furthermore, this public string Name { get; set; }
is equivalent to the following:
private string name;
public string Name
{
get { return name; }
set { name = value; }
}
Comparing one another the first one does the same job with fewer lines of code. However, in both cases C# compiler will create as I stated above one method for setting the value and one method for getting the value. The main difference, it is that in the second case, the creation of backing field isn't needed.
Update
In order to be more clear the above , I created a console application, in which I added a class called Customer
with the following definition:
class Customer
{
public string Name { get; set; }
}
I run my fantastic application and then I looked at the MSIL code that had been created by the C#.
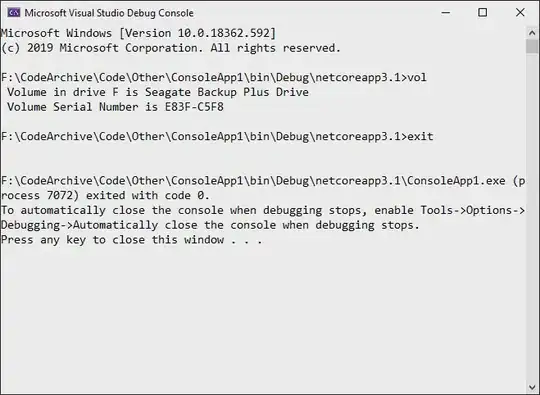
I created the above using a free .net disassembler, called Ildasm.exe (IL Disassembler). This tool as it's name implies shows the IL code C# compilers creates, when we build one application. A good tutorial about this tool can be found here.
As you notice the type Customer is compiled to a class that has a backing field and two methods, get_Name
and the set_Name
. These have been created automatically by the C# compiler.