How about:
subplot(numel(YourCell), 1, 1), imshow(YourCell{1});
for k=2:5
subplot(1,numel(YourCell),k), imshow(YourCell{k})
xlim([1 size(YourCell{1},1)]);
ylim([1 size(YourCell{1},2)]);
end
Result (with dummy data):
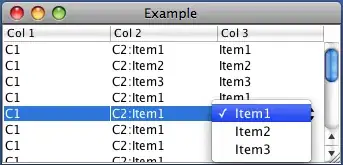
Edit:
You can play with the arrangement of your tiles by calculating the position of the next one. Here is a quick and dirty example, you can surely do a better job:
Side by side:
border=5;
MergedImage=ones(size(YourCell{1},1), 2.5*size(YourCell{1},2));
MergedImage(1:size(YourCell{1},1), 1:size(YourCell{1},2))=YourCell{1};
Pos=[1, size(YourCell{1},1)+border];
for k=1:(numel(YourCell)-1)
MergedImage(Pos(1):Pos(1)+size(YourCell{k+1}, 1)-1, Pos(2):Pos(2)+size(YourCell{k+1}, 2)-1)=YourCell{k+1};
Pos=[Pos(1), Pos(2)+size(YourCell{k+1}, 2)+border];
end
imshow(MergedImage);

Or a tighter arrangement:
border=5;
MergedImage=ones(size(YourCell{1},1), 2*size(YourCell{1},2));
MergedImage(1:size(YourCell{1},1), 1:size(YourCell{1},2))=YourCell{1};
Pos=[1, size(YourCell{1},1)+border];
for k=1:(numel(YourCell)-1)
MergedImage(Pos(1):Pos(1)+size(YourCell{k+1}, 1)-1, Pos(2):Pos(2)+size(YourCell{k+1}, 2)-1)=YourCell{k+1};
if mod(k,2) == 0
Pos=[Pos(1)+size(YourCell{k+1}, 1)+border, Pos(2)];
else
Pos=[Pos(1), Pos(2)+size(YourCell{k+1}, 2)+border];
end
end
imshow(MergedImage);
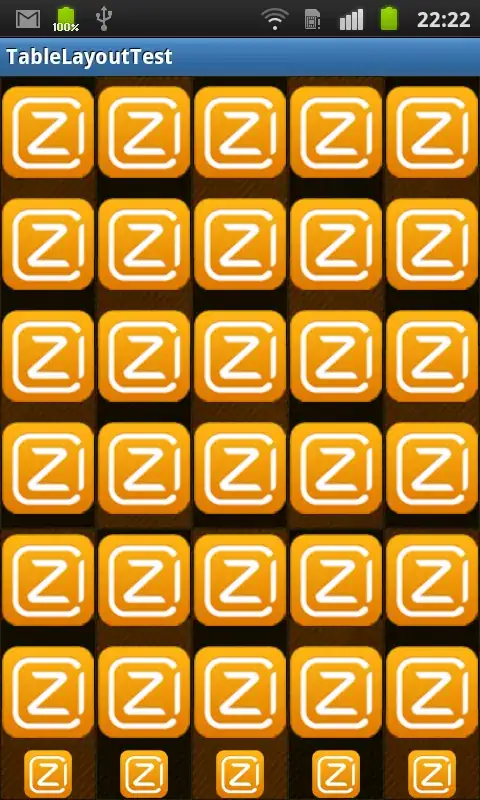