If I understand correctly, basically you want to change the color each time the button is pressed, based on the number of times the button has previously been pressed.
MouseListener
is not a good choice for JButton
, as buttons can be activated by the keyboard (via short cuts or focus activity) and programically, none of which the MouseListener
will detect.
Instead, you should use a ActionListener
This example will change the color each time the button is clicked. I've used an array of Color
s to make life simpler, but the general concept should work for if-else
statements, you just need to reset the counter when it reaches its limit
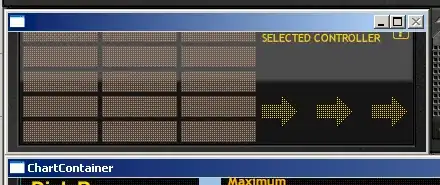
import java.awt.Color;
import java.awt.Dimension;
import java.awt.EventQueue;
import java.awt.GridBagLayout;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.UIManager;
import javax.swing.UnsupportedLookAndFeelException;
public class ButtonClicker {
public static void main(String[] args) {
new ButtonClicker();
}
public ButtonClicker() {
EventQueue.invokeLater(new Runnable() {
@Override
public void run() {
try {
UIManager.setLookAndFeel(UIManager.getSystemLookAndFeelClassName());
} catch (ClassNotFoundException | InstantiationException | IllegalAccessException | UnsupportedLookAndFeelException ex) {
ex.printStackTrace();
}
JFrame frame = new JFrame("Testing");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.add(new TestPane());
frame.pack();
frame.setLocationRelativeTo(null);
frame.setVisible(true);
}
});
}
public static class TestPane extends JPanel {
private static final Color[] COLORS = new Color[]{Color.ORANGE, Color.RED, Color.BLACK};
private int clickCount;
public TestPane() {
setLayout(new GridBagLayout());
JButton clicker = new JButton("Color Changer");
clicker.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
clickCount++;
setBackground(COLORS[Math.abs(clickCount % COLORS.length)]);
}
});
setBackground(COLORS[clickCount]);
add(clicker);
}
@Override
public Dimension getPreferredSize() {
return new Dimension(200, 200);
}
}
}
Take a look at How to Use Buttons, Check Boxes, and Radio Buttons and How to Write an Action Listeners for more details