Based on @Yury Tarabanko (that didn't work for me, but gave me an inspiration), here is what I got:
//Global Object where perfomance reuslts will be stored
perf = {};
Then, you need a function that wraps all other functions and tracks perfomance.
//Function that actually tracks the perfomance, wrapping all other functions
function trackPerfomance() {
var name, fn;
for (name in jNTRender) { //jNTRender - is the namespace that I was analysing. Use yours or window
fn = jNTRender[name];
if (typeof fn === 'function') {
jNTRender[name] = (function(name, fn) {
var args = arguments;
return function() {
if (!perf[name]) perf[name] = {
timesCalled: 0,
timeTaken: 0,
averageTime: 0
}
var start = performance.now(),
out = fn.apply(this, arguments),
end = performance.now();
perf[name].timesCalled ++; //how many times function was called
perf[name].timeTaken += (end - start); //time taken for execution
perf[name].averageTime = perf[name].timeTaken/perf[name].timesCalled; //average execution time
return out;
}
})(name, fn);
}
}
}
And you need to analyse results...
//Function that analyzes results - jQuery used for simplicity
function analyzePerfomance(){
functionsAverageTime = [];
$.each(jNTPerfomance, function(functionName, functionPerfomance){
functionsAverageTime.push([functionName, functionPerfomance.averageTime]);
});
functionsAverageTime.sort(function(a, b) { return b[1]-a[1] });
console.log('Slowest in average functions, msec');
$.each(functionsAverageTime, function(index, value){
console.log(index+1, value[0], value[1]);
});
functionsTimesCalled = [];
$.each(jNTPerfomance, function(functionName, functionPerfomance){
functionsTimesCalled.push([functionName, functionPerfomance.timesCalled]);
});
console.log('Most used functions, times');
$.each(functionsTimesCalled, function(index, value){
console.log(index+1, value[0], value[1]);
});
functionsTotalTimeSpent = [];
totalTime = 0;
$.each(jNTPerfomance, function(functionName, functionPerfomance){
totalTime += functionPerfomance.timeTaken;
});
$.each(jNTPerfomance, function(functionName, functionPerfomance){
functionsTotalTimeSpent.push([functionName, functionPerfomance.timeTaken, 100*functionPerfomance.timeTaken/totalTime]);
});
functionsTotalTimeSpent.sort(function(a, b) { return b[1]-a[1] });
console.log('Time taken by functions, msec, % of total time taken');
$.each(functionsTotalTimeSpent, function(index, value){
console.log(index+1, value[0], Math.round(value[1]), value[2].toFixed(2) + '%');
});
}
Run the tracker from console.
trackPerfomance();
Wait for some time - minutes, hours...
And analize perfomance:
analyzePerfomance();
Here is what I get in console. Really usefull, easy to read. And of cource it's possible to send perf object via ajax to server.
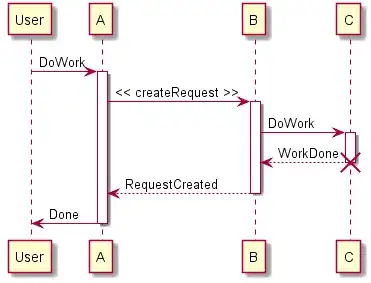