My suggestion for your case is use Button
initially set text and set gravity
(not layout_gravity) to left|center_vertical
instead of opening an AlertDialog
open a PopupWindow
set that Button as anchor of that PopUpWindow. In that PopUpWindow
place a ListView
and in OnItemClick
change text with selected value in that Button using setText(java.lang.CharSequence)
code snippet
XML for that Button
<Button
android:id="@+id/propertyBtn"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginRight="@dimen/dp_4"
android:background="@drawable/property_btn_large"
android:ellipsize="marquee"
android:gravity="left|center_vertical"
android:marqueeRepeatLimit="marquee_forever"
android:minHeight="0dp"
android:minWidth="0dp"
android:onClick="showPropertyPopUp"
android:paddingLeft="42dp"
android:paddingRight="22dp"
android:shadowColor="@android:color/white"
android:shadowDx="1"
android:shadowDy="1"
android:shadowRadius="1"
android:text="@string/select_property" />
Java code for opening PopUpWindow
in that Button click
//don't forget to initialize that button in onCreate(...)
public void showPropertyPopUp(View v) {
propertyList = dbHelper.getAllProperties();
dbHelper.closeDB();
if(propertyList != null && propertyList.size() > 0) {
LayoutInflater inflater = (LayoutInflater) getSystemService(Context.LAYOUT_INFLATER_SERVICE);
View popUpView = inflater.inflate(R.layout.pop_up, null, false);
Bitmap bitmap = BitmapFactory.decodeResource(getResources(),
R.drawable.select_dropdown);
popupWindowProperty = new PopupWindow(popUpView, propertyBtn.getWidth(),
300, true);
popupWindowProperty.setContentView(popUpView);
popupWindowProperty.setBackgroundDrawable(new BitmapDrawable(getResources(),
bitmap));
popupWindowProperty.setOutsideTouchable(true);
popupWindowProperty.setFocusable(true);
popupWindowProperty.showAsDropDown(propertyBtn, 0, 0);
ListView dropdownListView = (ListView) popUpView.
findViewById(R.id.dropdownListView);
PropertyDropdownAdapter adapter = new PropertyDropdownAdapter(
AddIncomeActivity.this,
R.layout.row_pop_up_list, propertyList);
dropdownListView.setAdapter(adapter);
dropdownListView.setOnItemClickListener(this);
}
}
code for setting text on that Button
in OnItemClick
PropertyInfo addPropertyInfo = propertyList.get(position);
String propertyName = addPropertyInfo.getPropertyName();
propertyBtn.setText(propertyName);
popupWindowProperty.dismiss();
pop_up layout
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical" >
<ListView
android:id="@+id/dropdownListView"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:cacheColorHint="@null"
android:fadeScrollbars="false" >
</ListView>
</LinearLayout>
Screenshot on clicking on that Button
Screenshot after item click of ListView
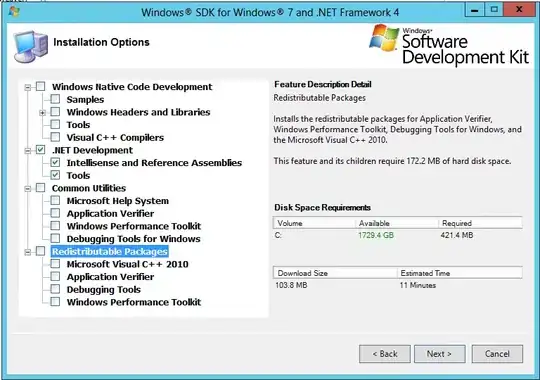