You should really look into implementing a MVVM pattern, in most cases you won't need to reference any buttons since it would be part of your ViewModel and you can loop through the collection that you want to change.
However, given your information you have something like this 3x3 with buttons in each one?
<Grid x:Name="myGrid">
<Grid.ColumnDefinitions>
<ColumnDefinition Width="33*"/>
<ColumnDefinition Width="33*"/>
<ColumnDefinition Width="33*"/>
</Grid.ColumnDefinitions>
<Grid.RowDefinitions>
<RowDefinition Height="33*"/>
<RowDefinition Height="33*"/>
<RowDefinition Height="33*"/>
</Grid.RowDefinitions>
<Button Content="1" Grid.Row="0" Grid.Column="0" ></Button>
<Button Content="2" Grid.Row="0" Grid.Column="1" ></Button>
<Button Content="3" Grid.Row="0" Grid.Column="2" ></Button>
<Button Content="4" Grid.Row="1" Grid.Column="0" ></Button>
<Button Content="5" Grid.Row="1" Grid.Column="1" ></Button>
<Button Content="6" Grid.Row="1" Grid.Column="2" ></Button>
<Button Content="7" Grid.Row="2" Grid.Column="0" ></Button>
<Button Content="8" Grid.Row="2" Grid.Column="1" ></Button>
<Button Content="9" Grid.Row="2" Grid.Column="2" ></Button>
</Grid>
Then in the code behind is very simple foreach
by going through the Grid's children
foreach (Button b in this.myGrid.Children)
{
// do whatever you want with b
// b.Content = "I'm a button";
}
You can also .Tag each button which a specific identifier and even loop them as well
foreach (Button b in this.myGrid.Children)
{
if(b.Tag == "main_buttons")
{
// do whatever you want with b when b.Tag is what you want
}
}
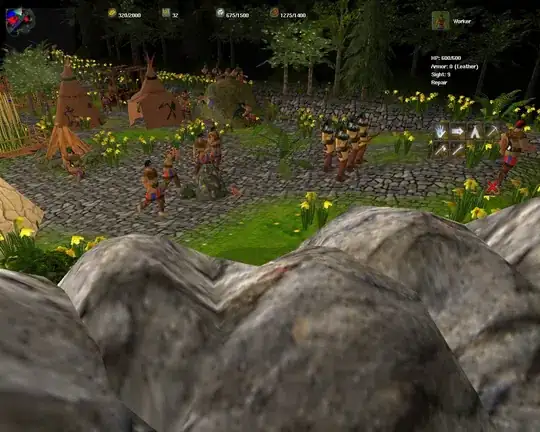