I dare your teacher to make that layout work on MacOS, Linux, Solaris, Windows 7 and Windows 8 without it look (even) more crap
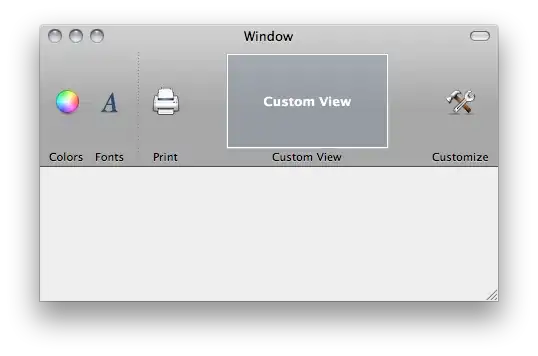
import java.awt.EventQueue;
import java.awt.GridBagConstraints;
import java.awt.GridBagLayout;
import java.awt.Insets;
import javax.swing.JButton;
import javax.swing.JCheckBox;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JPanel;
import javax.swing.JScrollBar;
import javax.swing.JScrollPane;
import javax.swing.JTextArea;
import javax.swing.JTextField;
import javax.swing.UIManager;
import javax.swing.UnsupportedLookAndFeelException;
import javax.swing.border.EmptyBorder;
public class TestLayout40 {
public static void main(String[] args) {
new TestLayout40();
}
public TestLayout40() {
EventQueue.invokeLater(new Runnable() {
@Override
public void run() {
try {
UIManager.setLookAndFeel(UIManager.getSystemLookAndFeelClassName());
} catch (ClassNotFoundException | InstantiationException | IllegalAccessException | UnsupportedLookAndFeelException ex) {
ex.printStackTrace();
}
JFrame frame = new JFrame("Testing");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.add(new TestPane());
frame.pack();
frame.setLocationRelativeTo(null);
frame.setVisible(true);
}
});
}
public class TestPane extends JPanel {
public TestPane() {
setLayout(new GridBagLayout());
setBorder(new EmptyBorder(8, 8, 8, 8));
GridBagConstraints gbc = new GridBagConstraints();
gbc.gridx = 0;
gbc.gridy = 0;
gbc.gridwidth = 2;
gbc.ipadx = 30;
gbc.ipady = 30;
gbc.anchor = GridBagConstraints.LINE_START;
add(new JButton("Button"), gbc);
gbc = new GridBagConstraints();
gbc.gridx = 2;
gbc.gridy = 0;
gbc.gridwidth = 2;
gbc.ipady = 30;
gbc.anchor = GridBagConstraints.LINE_START;
gbc.insets = new Insets(0, 15, 0, 0);
add(new JTextField("TextField", 15), gbc);
gbc = new GridBagConstraints();
gbc.gridx = 0;
gbc.gridy = 1;
add(new JScrollBar(), gbc);
gbc = new GridBagConstraints();
gbc.gridx = 1;
gbc.gridy = 1;
gbc.gridwidth = 2;
gbc.ipadx = 30;
gbc.fill = GridBagConstraints.BOTH;
gbc.insets = new Insets(15, 0, 0, 0);
add(new JScrollPane(new JTextArea(5, 10)), gbc);
gbc = new GridBagConstraints();
gbc.gridx = 1;
gbc.gridy = 2;
gbc.gridwidth = 2;
gbc.weighty = 1.0;
add(new JLabel("label"), gbc);
gbc = new GridBagConstraints();
gbc.gridx = 3;
gbc.gridy = 1;
gbc.anchor = GridBagConstraints.LINE_START;
gbc.weightx = 1.0;
add(new JCheckBox("Checkbox"), gbc);
}
}
}