There's no native or universal way as far as I know. I prefer to write .clone
method for my pseudo classes and use it:
Person.prototype.clone = function() {
var clone = new Person()
clone.name = this.name;
}
You could loop through own properties of the object to clone them while not copying stuff inherited from other objects:
for(var i in this) {
if(this.hasOwnProperty(i)) {
clone[i] = this[i];
}
}
This will copy everything that was added to your object after instantiating (eg. won't copy the prototype properties which were already added by calling new Person
.
thanks to Bergi
This method allows you to retrieve the prototype of any object. So instead of new Name
you can use:
var clone = Object.create(Object.getPrototypeOf(this))
That's an universal solution which can save you lot of work. You must then proceed with copying own properties using for(var i in ...)
.
Finally, you could create a person that inherits from the original object:
var clone = Object.create(this);
That can be useful in special cases, but normally I wouldn't recommend it.
It's also interesting to know that you can (in certain browsers firefox only) override method .toSource
which is defined for all objects, even the native ones:
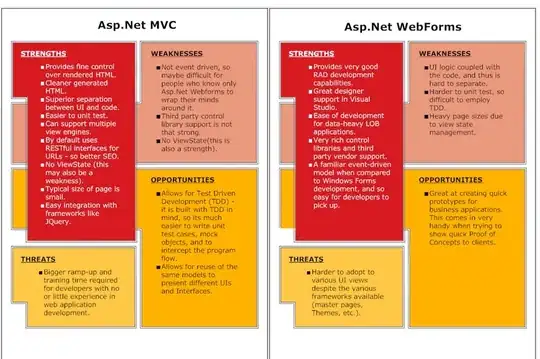
In certain cases, you might want to do:
Person.prototype.toSource = function() {
//Assuming name can be given in constructor
return "new Person("+this.name.toSource()+")";
}
Further reading
Aside from the linked docs, I found this nice article about javascript object inheritance: