Using a DataContractSerializer you tag those things you want to serialize with the appropriate Attribute. You did not share what you tried or how it failed when you tried to use it, but here is how:
Imports System.Runtime.Serialization
Imports System.ComponentModel
<DataContract>
Public Class Employee
<DataMember(Name:="Name")>
Public Property Name As String
<DataMember(Name:="Dept")>
Public Property Dept As String
<DataMember(Name:="Index")>
Public Property Index As Integer
<DataMember(Name:="secret")>
Private secret As Integer
' most serializers require a simple ctor
Public Sub New()
End Sub
Public Sub SetValue(n As Integer)
secret = n
End Sub
End Class
Use <DataContract>
on the class and <Datamember>
on the members. Then serializing:
' test data
Dim emp As New Employee With {.Name = "Ziggy", .Dept = "Manager", .Index = 2}
emp.SetValue(4) ' set private member value
Dim ser = New DataContractSerializer(GetType(Employee))
Using fs As New FileStream("C:\Temp\DCTest.xml", FileMode.OpenOrCreate),
xw = XmlWriter.Create(fs)
ser.WriteObject(xw, emp)
End Using
Output:
<?xml version="1.0" encoding="utf-8"?>
<Employee xmlns:i="..." xmlns="...">
<Dept>Manager</Dept>
<Index>2</Index>
<Name>Ziggy</Name>
<secret>4</secret>
</Employee>
As for one-size-fits-all functions for this type of thing, I personally tend to treat serialization as class or app or task specific (I also favor binary serialization over XML 100 to 1), but...
Private Function SerializeToString(Of T)(obj As T) As String
' there might be more efficient ways...
' this is off the top of my head
Dim ser = New DataContractSerializer(GetType(T))
Dim xml As String
Using ms As New MemoryStream()
ser.WriteObject(ms, obj)
ms.Position = 0
Using sr As New StreamReader(ms)
xml = sr.ReadToEnd
End Using
End Using
Return xml
End Function
Public Function DeserializeFromString(Of T)(xmlString As String) As T
Dim ser = New DataContractSerializer(GetType(T))
Dim ret As T
Using sr As New StringReader(xmlString),
ms As New MemoryStream(Encoding.Default.GetBytes(sr.ReadToEnd))
ret = CType(ser.ReadObject(ms), T)
End Using
Return ret
End Function
Seems to work in a round trip test:
Dim emp As New Employee With {.Name = "Ziggy", .Dept = "Manager", .Index = 2}
emp.SetValue(4)
Dim str = SerializeToString(Of Employee)(emp)
Dim emp2 As Employee = DeserializeFromString(Of Employee)(str)
' serialize to disk (not shown)
If SerializeToFile("C:\Temp\DCTest.xml", emp) Then
Dim emp3 As Employee = DeserializeFromFile(Of Employee)("C:\Temp\DCTest.xml")
End If
The deserialized version has the correct private, secret value:
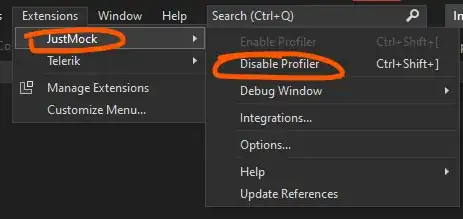