Do you have the outline paths of the heart, lungs, etc?
If yes, you just use context.isPointInPath
to hit-test each of your organs.
If no, you can draw each organ onto its own canvas and then use context.getImageData
to hit-test whether the pixel under the mouse is opaque for any particular organ-canvas.
Here's an example of hit-testing with the pixel data:
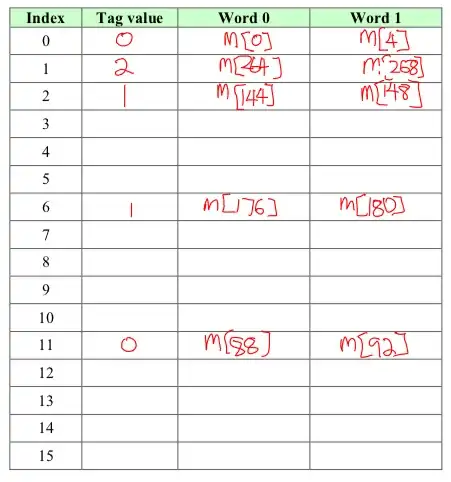
Highlighting the organ the mouse is hovering:
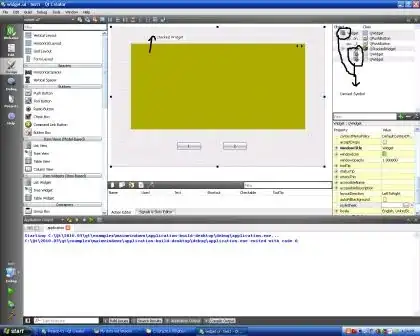
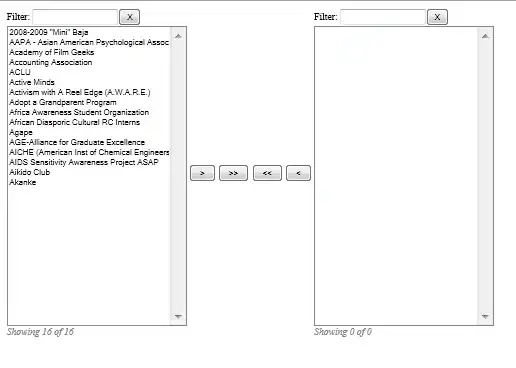
var canvas=document.getElementById("canvas");
var ctx=canvas.getContext("2d");
var cw=canvas.width;
var ch=canvas.height;
var $canvas=$("#canvas");
var canvasOffset=$canvas.offset();
var offsetX=canvasOffset.left;
var offsetY=canvasOffset.top;
var heartCanvas=document.createElement('canvas');
var heartCtx=heartCanvas.getContext('2d');
var lungsCanvas=document.createElement('canvas');
var lungsCtx=lungsCanvas.getContext('2d');
heartCanvas.width=lungsCanvas.width=canvas.width;
heartCanvas.height=lungsCanvas.height=canvas.height;
var heart={canvas:heartCanvas,x:100,y:125,alpha:0.25};
var lungs={canvas:lungsCanvas,x:65,y:105,alpha:0.25};
var imgCount=3;
var bodyImg=new Image();bodyImg.onload=start;bodyImg.src="https://dl.dropboxusercontent.com/u/139992952/multple/humanOutline.png";
var heartImg=new Image();heartImg.crossOrigin='anonymous';heartImg.onload=start;heartImg.src="https://dl.dropboxusercontent.com/u/139992952/multple/heart.png";
var lungsImg=new Image();lungsImg.crossOrigin='anonymous';lungsImg.onload=start;lungsImg.src="https://dl.dropboxusercontent.com/u/139992952/multple/lungs.png";
function start(){
if(--imgCount>0){return;}
var data;
heartCtx.drawImage(heartImg,heart.x,heart.y);
data=heartCtx.getImageData(0,0,cw,ch).data;
heart.pixels=[];
for(var i=0;i<data.length;i+=4){heart.pixels.push(data[i+3]);}
lungsCtx.drawImage(lungsImg,lungs.x,lungs.y);
data=lungsCtx.getImageData(0,0,cw,ch).data;
lungs.pixels=[];
for(var i=0;i<data.length;i+=4){lungs.pixels.push(data[i+3]);}
$("#canvas").mousemove(function(e){handleMouseMove(e);});
draw();
}
function draw(){
ctx.clearRect(0,0,cw,ch);
ctx.globalAlpha=0.25;
ctx.drawImage(bodyImg,0,0);
ctx.globalAlpha=heart.alpha;
ctx.drawImage(heartCanvas,0,0);
ctx.globalAlpha=lungs.alpha;
ctx.drawImage(lungsCanvas,0,0);
ctx.globalAlpha=1.00;
}
function handleMouseMove(e){
// tell the browser we're handling this event
e.preventDefault();
e.stopPropagation();
mouseX=parseInt(e.clientX-offsetX);
mouseY=parseInt(e.clientY-offsetY);
var heartPixelAlpha=heart.pixels[mouseY*cw+mouseX];
heart.alpha=(heartPixelAlpha>0)?1.00:0.25;
var lungsPixelAlpha=lungs.pixels[mouseY*cw+mouseX];
lungs.alpha=(lungsPixelAlpha>0)?1.00:0.25;
draw();
}
body{ background-color: ivory; }
#canvas{border:1px solid red;}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/1.9.1/jquery.min.js"></script>
<h4>Hover mouse over 1+ organs to highlight them</h4>
<canvas id="canvas" width=300 height=330></canvas>