You "style" XML using Extensible Stylesheet Language Transformations (XSLT). XSLT parses XML into HTML using stylesheets. You can then use standard CSS to format the display of the resulting HTML.
For the sample XML you cited, this stylesheet:
<?xml version="1.0" encoding="utf-8"?>
<xsl:stylesheet xmlns:xsl="http://www.w3.org/1999/XSL/Transform" version="1.0">
<xsl:output method="html"/>
<xsl:template match="/">
<html>
<body>
<xsl:for-each select="note">
<p> <xsl:value-of select="body"/> </p>
</xsl:for-each>
</body>
</html>
</xsl:template>
</xsl:stylesheet>
would give you a properly formed HTML document that just listed the note
body. EG: Don't forget me this weekend!
from your example.
Use XSLTProcessor()
to run XSLT in a Greasemonkey script, like so:
// ==UserScript==
// @name _XML renderer / styler
// @description stylesheet for xml results
// @include http://YOUR_SERVER.COM/YOUR_PATH/*.xml
// @include http://www.w3schools.com/xml/note.xml
// @grant none
// ==/UserScript==
//-- Warning, multiline str is new in JS. Only Firefox supports at the moment.
var xsl_str = `<?xml version="1.0" encoding="utf-8"?>
<xsl:stylesheet xmlns:xsl="http://www.w3.org/1999/XSL/Transform" version="1.0">
<xsl:output method="html"/>
<xsl:template match="/">
<html>
<head>
<title>My super new note format!</title>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
<style type="text/css">
body { padding: 0 2em; }
.noteCtr {
border: 1px solid gray;
border-radius: 1ex;
padding: 0;
background: #FAFAFA;
}
.messPeople { font-size: 1em; margin: 1ex 1em; }
.messHeading { background: lightcyan; margin: 0 1.6ex; }
.messHeading::after { content: ":"; }
.noteCtr > p {
background: white;
padding: 1em;
margin: 0 1em 1.5ex 1em;
}
</style>
</head>
<body>
<xsl:for-each select="note">
<div class="noteCtr">
<h3 class="messPeople">
<xsl:value-of select="from"/>
-->
<xsl:value-of select="to"/>
</h3>
<h3 class="messHeading"> <xsl:value-of select="heading"/> </h3>
<p> <xsl:value-of select="body"/> </p>
</div>
</xsl:for-each>
</body>
</html>
</xsl:template>
</xsl:stylesheet>
`;
var processor = new XSLTProcessor ();
var dataXSL = new DOMParser ().parseFromString (xsl_str, "text/xml");
processor.importStylesheet (dataXSL);
var newDoc = processor.transformToDocument (document);
//-- These next lines swap the new, processed doc in for the old one...
window.content = newDoc;
document.replaceChild (
document.importNode (newDoc.documentElement, true),
document.documentElement
);
Here I stored the stylesheet in the xsl_str
variable. For more involved operations, you may find it better to fetch the stylesheet via a @resource
directive.
Install that script and then visit your example XML.
You will see it transform that document...
From:
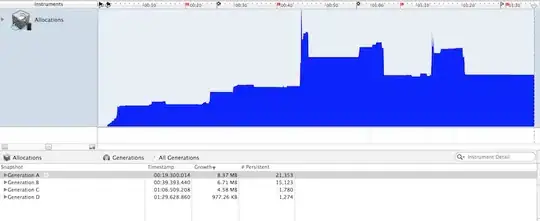
To:
