How about the DataGridView.EditMode
Property which
Gets or sets a value indicating how to begin editing a cell.
where
The default is EditOnKeystrokeOrF2
.
and
All DataGridViewEditMode
values except for EditProgrammatically
allow a user to double-click a cell to begin editing it.
You have several options to choose from the DataGridViewEditMode
Enumeration
EditOnEnter
- Editing begins when the cell receives focus. This mode is useful when pressing the TAB key to enter values across a row, or when pressing the ENTER key to enter values down a column.
EditOnF2
- Editing begins when F2 is pressed while the cell has focus. This mode places the selection point at the end of the cell contents.
EditOnKeystroke
- Editing begins when any alphanumeric key is pressed while the cell has focus.
EditOnKeystrokeOrF2
- Editing begins when any alphanumeric key or F2 is pressed while the cell has focus.
EditProgrammatically
- Editing begins only when the BeginEdit method is called.
Update for DataGridViewCheckBoxCell
:
It turns out that the DataGridViewEditMode
does not work for the DataGridViewCheckBoxColumn
.
In this case you can create your own DataGridViewCheckBoxColumn
& DataGridViewCheckBoxCell
. This allows you to override the cell's OnKeyUp
event handler and reset the EditingCellFormattedValue
if Space
was pressed.
public class MyCheckBoxColumn : DataGridViewCheckBoxColumn
{
public MyCheckBoxColumn()
{
CellTemplate = new MyCheckBoxCell();
}
}
public class MyCheckBoxCell : DataGridViewCheckBoxCell
{
protected override void OnKeyUp(KeyEventArgs e, int rowIndex)
{
if (e.KeyCode == Keys.Space)
{
e.Handled = true;
if (EditingCellValueChanged)
{
// Reset the value.
EditingCellFormattedValue = !(bool)EditingCellFormattedValue;
}
}
else
{
base.OnKeyUp(e, rowIndex);
}
}
}
After you rebuild your project the new column should appear in the designer:
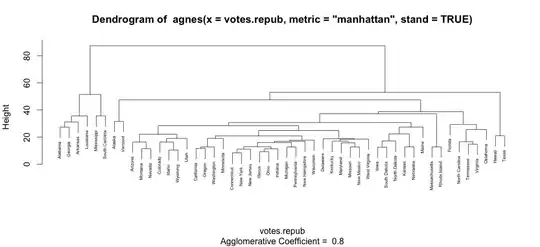