Keeping Bootstrap's navbar-top-fixed
from zooming on mobiles
The final result:
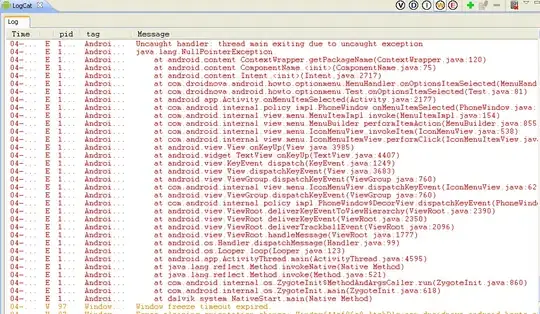
On mobile devices only, is possible to calculate the zoom factor as the ratio of window.innerWidth
and the screen width, as shown in [2]. When the user toggles zoom, the resize event is triggered; moreover, when a page is reloaded that was previously zoomed, the zoom factor is maintained. Thus, one use jQuery to dynamically update CSS transforms that keep the header in shape. Note that, with position: fixed
, a transformation of the origin is also required. Applying class device-fixed-height
to the bootstrap nav
and device-fixed-width
to brand logo and hamburger icon then produce very close to the desired result.
- Bug: As the page is zoomed, the hamburger icons still moves a bit to the left. Any suggestions how to fix it are appreciated!
- Tested on: Android 4.4
Live example: http://www.exploretrade.info/other/example-fixed-nav-after-zoom.html
Source code:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1">
<meta name="description" content="">
<meta name="author" content="">
<link rel="icon" href="../../favicon.ico">
<title>Bootstrap zoom-proof fixed top nav</title>
<!-- Bootstrap core CSS -->
<link href="//maxcdn.bootstrapcdn.com/bootstrap/3.3.1/css/bootstrap.min.css" rel="stylesheet">
<!-- Custom styles for this template -->
<link href="//maxcdn.bootstrapcdn.com/font-awesome/4.2.0/css/font-awesome.min.css" rel="stylesheet">
<style>
body {
padding-top: 50px;
}
</style>
</head>
<body>
<nav class="navbar navbar-inverse navbar-fixed-top device-fixed-height">
<div class="container">
<div class="navbar-header">
<button type="button" class="navbar-toggle collapsed device-fixed-width" data-toggle="collapse" data-target="#navbar" aria-expanded="false" aria-controls="navbar">
<span class="sr-only">Toggle navigation</span>
<i class="fa fa-bars"></i>
</button>
<a class="navbar-brand device-fixed-width" href="#">Example</a>
</div>
<div id="navbar" class="collapse navbar-collapse device-fixed-width">
<ul class="nav navbar-nav">
<li class="active"><a href="#">Home</a></li>
<li><a href="#about">About</a></li>
<li><a href="#contact">Contact</a></li>
</ul>
</div><!--/.nav-collapse -->
</div>
</nav>
<div class="container">
<div class="starter-template">
<h1>Bootstrap starter template</h1>
<p class="lead">Use this document as a way to quickly start any new project.<br> All you get is this text and a mostly barebones HTML document.</p>
<p>Lorem ipsum dolor sit amet, consectetur adipiscing elit. In in metus eget nisi imperdiet dictum ac eu metus. Morbi consequat sodales porta. Nam convallis sed dolor in ullamcorper. Vestibulum ut tortor porttitor, venenatis nulla iaculis, sollicitudin metus. Mauris ut hendrerit purus, sed ultricies lacus. Proin imperdiet, lectus vel efficitur hendrerit, quam tortor efficitur sapien, vehicula viverra magna ipsum vitae lacus. Sed faucibus elit vel massa placerat, in porttitor est suscipit. Pellentesque consequat condimentum elit, at sagittis erat euismod nec. Fusce consequat purus quis turpis volutpat, vel luctus tortor consectetur. Sed in lectus vitae enim fringilla faucibus. Mauris vitae risus ut ex convallis luctus. Interdum et malesuada fames ac ante ipsum primis in faucibus. Etiam tempor ante augue, sed iaculis nisi porta quis.</p>
</div>
</div><!-- /.container -->
<!-- Bootstrap core JavaScript
================================================== -->
<!-- Placed at the end of the document so the pages load faster -->
<script src="//ajax.googleapis.com/ajax/libs/jquery/1.11.1/jquery.min.js"></script>
<script src="//maxcdn.bootstrapcdn.com/bootstrap/3.3.1/js/bootstrap.min.js"></script>
<script>
// from https://signalvnoise.com/posts/2407-device-scale-user-interface-elements-in-ios-mobile-safari
function getDeviceScale() {
var deviceWidth, landscape = Math.abs(window.orientation) == 90;
if (landscape) {
// iPhone OS < 3.2 reports a screen height of 396px
deviceWidth = Math.max(480, screen.height);
} else {
deviceWidth = screen.width;
}
return window.innerWidth / deviceWidth;
}
// mobile only - keep the position:fixed header at constant size when page is zoomed
if (navigator.userAgent.match(/Mobi/)) {
$(window).on('load scroll', function() {
var ds = getDeviceScale();
$('.device-fixed-height').css('transform','scale(1,' + ds + ')')
.css('transform-origin', '0 0');
$('.device-fixed-width').css('transform', 'scale(' + ds + ',1)')
.css('transform-origin', '0 0');
})
}
</script>
</script>
</body>
</html>