0. NotesRichTextRange.SetStyle method
NotesRichTextRange.SetStyle
method is what you are looking for. For this method you need to create NotesRichTextStyle
object. Also you need to SetBegin
end SetEnd
of range by using NotesRichTextNavigator
object.
Here is example:
Dim ses As New NotesSession
Dim doc As NotesDocument
Dim richText As NotesRichTextItem
Dim navigator As NotesRichTextNavigator
Dim range As NotesRichTextRange
Dim headerStyle As NotesRichTextStyle
Dim descriptionStyle As NotesRichTextStyle
Dim footerStyle As NotesRichTextStyle
'Create your doc.
'Generate rich text content:
Set richText = doc.CreateRichTextItem("Body")
Set navigator = richText.CreateNavigator
Set range = richText.CreateRange
richText.AppendText("Header")
richText.AddNewline(1)
Set headerStyle = ses.CreateRichTextStyle
headerStyle.Underline = True
Set descriptionStyle = ses.CreateRichTextStyle
descriptionStyle.Bold = True
Set footerStyle = ses.CreateRichTextStyle
footerStyle.NotesColor = COLOR_RED
navigator.FindFirstElement(RTELEM_TYPE_TEXTPARAGRAPH)
range.SetBegin(navigator)
range.SetEnd(navigator)
Call range.SetStyle(headerStyle)
For index% = 0 To 7
richText.AppendText("Description" & index%)
richText.AddNewline(1)
navigator.FindNextElement(RTELEM_TYPE_TEXTPARAGRAPH)
range.SetBegin(navigator)
range.SetEnd(navigator)
Call range.SetStyle(descriptionStyle)
Next
richText.AppendText("Footer")
richText.AddNewline(1)
navigator.FindNextElement(RTELEM_TYPE_TEXTPARAGRAPH)
range.SetBegin(navigator)
range.SetEnd(navigator)
Call range.SetStyle(footerStyle)
Call richText.EmbedObject(EMBED_ATTACHMENT, "", "SomeFile")
richText.Update
'Process your doc.
This example generates this rich text:
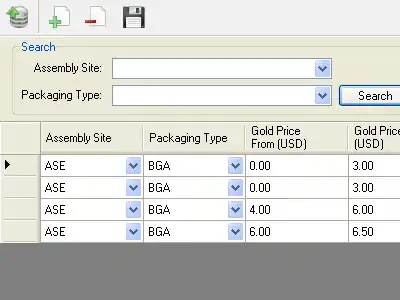
1. NotesDocument.RenderToRTItem method
The other way is to use NotesDocument.RenderToRTItem
method. For this method you need to create a form and style it as you need. For example, create a form "Message" and add to this form four fields:

And use this form in your code:
Dim ses As New NotesSession
Dim db As NotesDatabase
Dim messageDoc As NotesDocument
Dim attachment As NotesRichTextItem
Dim description(7) As String
Dim doc As NotesDocument
Dim richText As NotesRichTextItem
Set db = ses.CurrentDatabase
Set messageDoc = db.CreateDocument
messageDoc.Form = "Message"
messageDoc.Header = "Header"
For index% = 0 To Ubound(description)
description(index%) = "Description" & index%
Next
messageDoc.Description = description
messageDoc.Footer = "Footer"
Set attachment = messageDoc.CreateRichTextItem("Attachment")
Call attachment.EmbedObject(EMBED_ATTACHMENT, "", "SomeFile")
'Create your doc.
'Generate rich text content:
Set richText = doc.CreateRichTextItem("Body")
Call messageDoc.RenderToRTItem(richText)
richText.Update
'Process your doc.
This example generates this rich text:
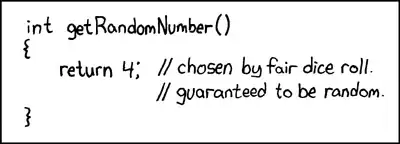
2. NotesUIDocument.Import method
You can genereate the rich text content somewhere else and import it to your document by using NotesUIDocument.Import
method.
Here is example for importing html
content:
Dim ses As New NotesSession
Dim db As NotesDatabase
Dim doc As NotesDocument
Dim richText As NotesRichTextItem
Dim ws As New NotesUIWorkspace
Dim uidoc As NotesUIDocument
'Generate html file
tempdir$ = Environ("Temp")
file = Freefile
filename$ = tempdir$ & "\temp.html"
Open filename$ For Output As file
Print #file, "<u>Header</u><br>"
For index% = 0 To 7
Print #file, "<b>Description" & index% & "</b><br>"
Next
Print #file, "<font color='red'>Footer</font><br><br>"
Close file
Set db = ses.CurrentDatabase
Set doc = db.CreateDocument
'Create your doc.
'Add attachment to rich text:
Set richText = doc.CreateRichTextItem("Body")
Call richText.EmbedObject(EMBED_ATTACHMENT, "", "SomeFile")
Set uidoc = ws.EditDocument(True, doc)
uidoc.GotoField("Body")
uidoc.Import "html", filename$
'Process your doc.
This example generates this rich text:
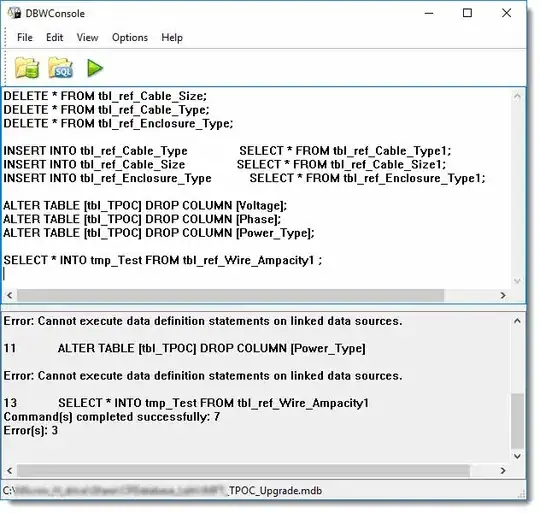