I tried installing django-cors-headers
to fix the error I was getting when running my django app with production settings.
' URL ' from origin 'http://localhost:3000' has been blocked by CORS policy: No 'Access-Control-Allow-Origin' header is present on the requested resource.
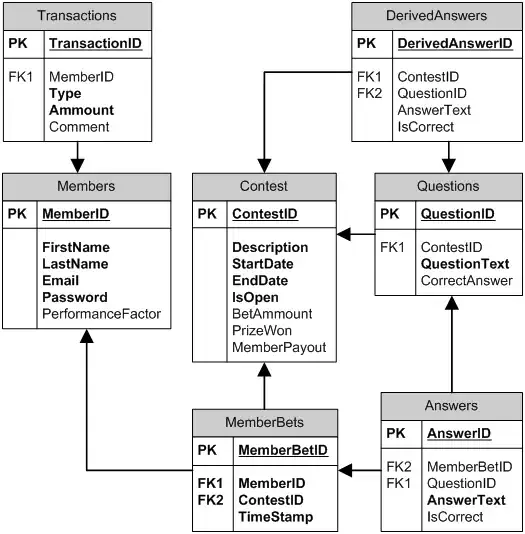
My app loads fine when running in development and installing django-cors-headers
did not resolve the issue. For some reason chrome was blocking Material
icons from loading when I was in production.
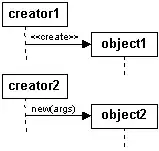
Once I discovered what the issue was I felt it was important to share how I fixed it as I feel a lot of people coming to this question will have the same circumstances.
This solution will hopefully work for those who are serving their static content (like images) from another server such as an AWS S3 bucket like I was and getting this error.
If you are and it is being blocked by chrome in the same way, installing django-cors-headers
won't do anything. This is because the problem lies with the configuration of the S3 bucket (Azure etc) and not the django app.
Go to the AWS S3 dashboard and once you select the bucket you are using to host the static files of your django app, click on the permissions tab.

Scroll down to the cross-origin resource sharing (CORS)
section and click 'Edit'.

And if you want to just resolve the issue completely (for just serving static files blocked by chrome), enter the following JSON and then click 'Save changes' at the bottom.
[
{
"AllowedHeaders": [],
"AllowedMethods": [
"GET"
],
"AllowedOrigins": [
"*"
],
"ExposeHeaders": []
}
]
There is more information here on how to configure your S3 bucket's CORS configuration.
After saving this configuration the error went away and my icons loaded properly.
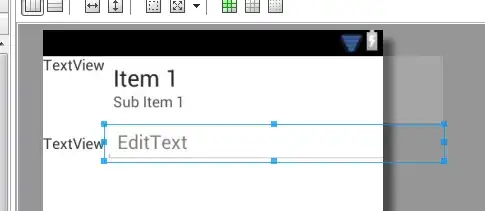
Footnote:
Given I was also misunderstanding how the Access-Control-Allow-Origin
header worked I have taken an image from Mozilla's Doc's on Cross-Origin Resource Sharing but edited it to show my situation to hopefully explain how the header works.
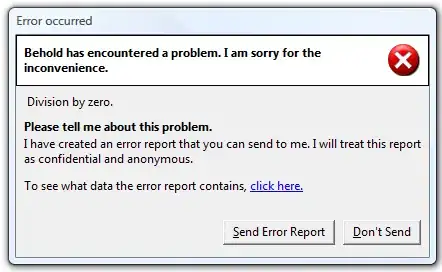
Imagine that Chrome and localhost were exchanging mail and all of a sudden chrome starts getting mail from AWS but it doesn't have Chromes name of it. Good guy Chrome is thinking, uh I don't know if I should be viewing this information, it isn't from the origin (localhost) so I don't know if I'm allowed and it could be sensitive information sent by mistake. Therefore I won't open it.
The Allow-Access-Control-Origin header is S3 writing on that mail 'it's ok, you (Chrome) have permission to view the information in this mail'.
django-cors-headers
is necessary for situations were you have an app hosted on a server A (origin) that is making requests to a django app on server B, that is not the origin. For example, if your django app was a rest api hosted on heroku and you had a react/angular app hosted on Azure that made requests to that api - then your django app would need it.
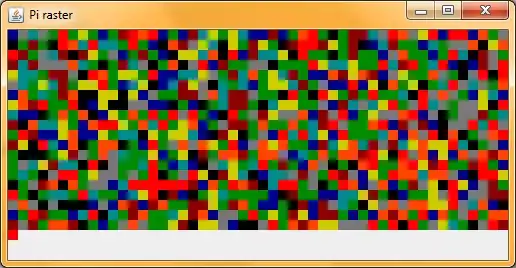