Solution using a ListView
with a custom ListAdapter
MainActivity.java:
public class MainActivity extends ListActivity implements AdapterView.OnItemClickListener {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
setUpComponents();
}
private void setUpComponents(){
ArrayList<String> myValuesToDisplay = getDatabaseContent();
MyCustomListAdapter adapter = new MyCustomListAdapter(this, myValuesToDisplay);
setListAdapter(adapter);
getListView().setOnItemClickListener(this);
}
private ArrayList<String> getDatabaseContent(){
/*
This is where you would like to connect to your database and fetch the content.
In this example, we simulate the result by returning an ArrayList<String>
*/
ArrayList<String> values = new ArrayList<String>();
values.add("Value1");
values.add("Value2");
values.add("Value3");
return values;
}
@Override
public void onItemClick(AdapterView<?> parent, View view, int position, long id) {
//When you click on an item in the list view, you fetch the position in the list
System.out.println("Clicked on item with position: " + position);
}
}
MyCustomListAdapter.java:
public class MyCustomListAdapter extends ArrayAdapter<String> {
private ArrayList<String> yourArray;
public MyCustomListAdapter(Context ctx, ArrayList<String> yourArray){
super(ctx, R.layout.my_custom_list_item, yourArray);
this.yourArray = yourArray;
}
@Override
public View getView(int position, View convertView, ViewGroup parent) {
//Re-use rows to save battery
View row;
if (convertView == null) {
//We inflate our custom view for the ListView item
LayoutInflater inflater = LayoutInflater.from(getContext());
row = inflater.inflate(
R.layout.my_custom_list_item, null);
} else {
row = convertView;
}
//Get the current item of the array
String arrayItem = yourArray.get(position);
//Get the text view in the layout of which we want to display the value
TextView tvListItem = (TextView) row.findViewById(R.id.tv_list_item);
//Set the text
tvListItem.setText(arrayItem);
//Return the row to the ListView
return row;
}
}
ActivityMain.xml:
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent"
android:layout_height="match_parent" android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
android:paddingBottom="@dimen/activity_vertical_margin" tools:context=".MainActivity">
<ListView
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:id="@android:id/list" />
</RelativeLayout>
my_custom_list_item.xml:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="New Text"
android:id="@+id/tv_list_item" />
</LinearLayout>
This solution will create a scrollable ListView
and populate it with your database values. Your implementation of the ListAdapter
can vary. You can choose what and how you would like to display by changing the layout in my_custom_list_item.xml
.
The result:
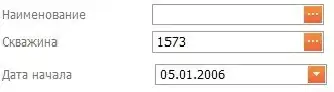
Clicking on a row will print out its position in the list. You can for example use that information to start another activity displaying detailed information about that entry.