Hard to give code when we don't know the format of the string...
...it might be a base 64 encoded string. Here's an example that shows the image in PictureBox1 being converted to a base 64 string, then back to an image and placed into PictureBox2:
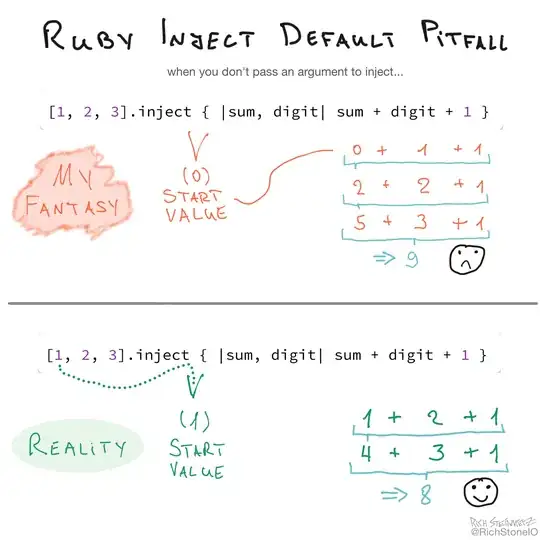
Dim oldMS As New System.IO.MemoryStream
PictureBox1.Image.Save(oldMS, System.Drawing.Imaging.ImageFormat.Jpeg)
Dim strData As String = Convert.ToBase64String(oldMS.ToArray)
Debug.Print(strData)
Dim bytes() As Byte = Convert.FromBase64String(strData) ' strData would come from your CSV file
Dim MS As New System.IO.MemoryStream(bytes)
Dim bmp As Image = Image.FromStream(MS)
PictureBox2.Image = bmp
Here's what the string looks like:
/9j/4AAQSkZJRgABAQEAYABgAAD/4QBORXhpZgAATU0AKgAAAAgABAMBAAUAAAABAAAAPlEQAAEAAAABAQAAAFERAAQAAAABAAAAAFESAAQAAAABAAAAAAAAAAAAAYagAACxj//bAEMACAYGBwYFCAcHBwkJCAoMFA0MCwsMGRITDxQdGh8eHRocHCAkLicgIiwjHBwoNyksMDE0NDQfJzk9ODI8LjM0Mv/bAEMBCQkJDAsMGA0NGDIhHCEyMjIyMjIyMjIyMjIyMjIyMjIyMjIyMjIyMjIyMjIyMjIyMjIyMjIyMjIyMjIyMjIyMv/AABEIACAAIAMBIgACEQEDEQH/xAAfAAABBQEBAQEBAQAAAAAAAAAAAQIDBAUGBwgJCgv/xAC1EAACAQMDAgQDBQUEBAAAAX0BAgMABBEFEiExQQYTUWEHInEUMoGRoQgjQrHBFVLR8CQzYnKCCQoWFxgZGiUmJygpKjQ1Njc4OTpDREVGR0hJSlNUVVZXWFlaY2RlZmdoaWpzdHV2d3h5eoOEhYaHiImKkpOUlZaXmJmaoqOkpaanqKmqsrO0tba3uLm6wsPExcbHyMnK0tPU1dbX2Nna4eLj5OXm5+jp6vHy8/T19vf4+fr/xAAfAQADAQEBAQEBAQEBAAAAAAAAAQIDBAUGBwgJCgv/xAC1EQACAQIEBAMEBwUEBAABAncAAQIDEQQFITEGEkFRB2FxEyIygQgUQpGhscEJIzNS8BVictEKFiQ04SXxFxgZGiYnKCkqNTY3ODk6Q0RFRkdISUpTVFVWV1hZWmNkZWZnaGlqc3R1dnd4eXqCg4SFhoeIiYqSk5SVlpeYmZqio6Slpqeoqaqys7S1tre4ubrCw8TFxsfIycrS09TV1tfY2dri4+Tl5ufo6ery8/T19vf4+fr/2gAMAwEAAhEDEQA/AOG8R69rvjTWdTvpbyVYoGYw24chVUdFUDjO0E++PeuS+13P/PxL/wB9muo8OXzrqF5YgxEmVp4lk4BZc5GexI5/4DjvWRrGkm3uZ5raJltwxJjPLQg9AfUe9ekopLQy5veszO+13P8Az8S/99mur8Pa9rngzW9OvIL6RklZfPt95KkHBKMDxnawPtn2rE0vTd08E91EzwlspAv358dh6D1J/Wr+sXcv261s5PKD+cLiVYjkBmxgbu5xzn/ax2FTJJqw09TFa4ktdVe4iOJI5iynHvXeeGYrDUDDNfTTJJGjTxWSRbmkReSqyZ4DYIww/wDr874v8I6t4a8QXVpdWkxjMjGGYISsik5BBHtWZaz6haxSQrFP5Mi7XUKQcexxx0FHM+X3WEopvU6XxAtlZ3V39keZyQJp7V02sFbBAaTPIGQMKBXJRzSXOqJNKcu8wZjj3qe7lv7pEiMM4hjAVEKk8D1Pc8n+mK0/CPhLVvEmv2tpaWk2zzFMszIQsag5JJ+naknaPvMdknof/9k=