You can use COUNT
to display your records and create pages
function getLimitData($start,$limit){
//getting all items
$db = new PDO('mysql:host=localhost; dbname=data','root','');
$results = $db->query("SELECT * FROM table ORDER BY ID DESC limit $start,$limit");
$results = $results->fetchAll(PDO::FETCH_ASSOC);
return $results;
}
//database connection
$db = new PDO('mysql:host=localhost; dbname=data','root','');
//pagination pages
$nav_counter = basename($_SERVER['SCRIPT_FILENAME']);
$page_counter = $nav_counter;
$nav_counter = rtrim($nav_counter, ".php");
$cPage = $page_counter + 1;
//creating next pages
$first_next = $nav_counter + 1 ;
$sec_next = $first_next + 1 ;
$third_next = $sec_next + 1 ;
$fourth_next = $third_next + 1 ;
$fifth_next = $fourth_next + 1 ;
$sixth_next = $fifth_next + 1 ;
$seventh_next = $sixth_next + 1 ;
$next_page = $seventh_next + 1;
//creating previous pages
$first_prev = $nav_counter - 1 ;
$sec_prev = $nav_counter - 2 ;
$third_prev = $nav_counter - 3 ;
$fourth_prev = $nav_counter - 4 ;
$fifth_prev = $nav_counter - 5 ;
$sixth_prev = $nav_counter - 6 ;
$seventh_prev = $nav_counter - 7 ;
//row count
$tableExists = $db->tableExist();
$ROW_COUNT = $db->getRowCount();
$numRows= 9; //number of items to be displayed
//last page
//last page
$last_page = ($ROW_COUNT / $numRows) - 1;
if(!is_int($last_page)){
$last_page = (int)$last_page + 1;
}
$pageNate = '';
if($ROW_COUNT <= $numRows){
$pageNate = 'class="hide"';
}else{
$pageNate = 'class="exist"';
}
//displaying torrents
$start = 0;
$limit = $numRows;
if ($page_counter !== 'index.php') {
$start = ($limit * $nav_counter);
}
//getting number of rows left in the table
$rows_left = $db->getLimitData($start, $limit);
$number_rows = 0;
foreach ($rows_left as $r) {
$number_rows = $number_rows + 1;
}
if ($number_rows < $numRows) {
$limit = $number_rows;
}
$items = $db->getLimitData($start, $limit);
?>
displaying item
<ol>
<?php foreach($items as $item) : ?>
<li><php echo $item['Value']; ?></li>
<?php endforeach; ?>
</ol>
pagination code
<?php
$pages = array();
for ($counter = 1; $counter <= $last_page; $counter++) {
$pages[] = $counter;
}
//storing pages in array and creating a page if it doesn't exist
foreach ($pages as $key) {
$page = $key.'.php';
//if page doesn't exists create page
if(file_exists($page)== false && $key <= $last_page){
copy('index.php', $page);
}
}
?>
<p class="pagenav" >
<a href="index.php" <?php if ($page_counter == 'index.php') {echo 'class="hide"';} ?>><<</a>
<a href="<?php if ($page_counter == '1.php') {echo 'index.php';}else{echo "$first_prev".".php";} ?>" <?php if ($page_counter == 'index.php') {echo 'class="hide"';} ?>><</a>
<a href="<?php echo "$seventh_prev".".php"; ?>" <?php if($seventh_prev <= 0){echo 'class="hide"';} ?>><?php echo $seventh_prev;?></a>
<a href="<?php echo "$sixth_prev".".php"; ?>" <?php if($sixth_prev <= 0){echo 'class="hide"';} ?>><?php echo $sixth_prev;?></a>
<a href="<?php echo "$fifth_prev".".php"; ?>" <?php if($fifth_prev <= 0){echo 'class="hide"';} ?>><?php echo $fifth_prev;?></a>
<a href="<?php echo "$fourth_prev".".php"; ?>" <?php if($fourth_prev <= 0){echo 'class="hide"';} ?>><?php echo $fourth_prev;?></a>
<a href="<?php echo "$third_prev".".php"; ?>" <?php if($third_prev <= 0){echo 'class="hide"';} ?>><?php echo $third_prev;?></a>
<a href="<?php echo "$sec_prev".".php"; ?>" <?php if($sec_prev <= 0){echo 'class="hide"';} ?>><?php echo $sec_prev;?></a>
<a href="<?php echo "$first_prev".".php"; ?>" <?php if($first_prev <= 0 ){echo 'class="hide"';} ?>><?php echo $first_prev;?></a>
<a <?php if ($page_counter == 'index.php') {echo 'class="hide"';}else{ echo 'id="here"';} ?>><?php echo $nav_counter; ?></a>
<a href="<?php echo $first_next.'.php'; ?>" <?php if($first_next <= $last_page){echo 'class="exist"';}else{echo 'class="hide"';} ?>><?php echo $first_next;?></a>
<a href="<?php echo "$sec_next".".php"; ?>" <?php if($sec_next <= $last_page){echo 'class="exist"';}else{echo 'class="hide"';} ?>><?php echo $sec_next;?></a>
<a href="<?php echo "$third_next".".php"; ?>" <?php if($third_next <= $last_page){echo 'class="exist"';}else{echo 'class="hide"';} ?>><?php echo $third_next;?></a>
<a href="<?php echo "$fourth_next".".php"; ?>" <?php if($fourth_next <= $last_page){echo 'class="exist"';}else{echo 'class="hide"';} ?>><?php echo $fourth_next;?></a>
<a href="<?php echo "$fifth_next".".php"; ?>" <?php if($fifth_next <= $last_page){echo 'class="exist"';}else{echo 'class="hide"';} ?>><?php echo $fifth_next;?></a>
<a href="<?php echo "$sixth_next".".php"; ?>" <?php if($sixth_next <= $last_page){echo 'class="exist"';}else{echo 'class="hide"';} ?>><?php echo $sixth_next;?></a>
<a href="<?php echo "$seventh_next".".php"; ?>" <?php if($seventh_next <= $last_page){echo 'class="exist"';}else{echo 'class="hide"';} ?>><?php echo $seventh_next;?></a>
<a href="<?php echo "$first_next".".php"; ?>" <?php if($first_next <= $last_page){echo 'class="exist"';}else{echo 'class="hide"';} ?>>></a>
<a href="<?php echo $last_page.'.php'; ?>" <?php if($nav_counter == $last_page){echo 'class="hide"';}else{echo 'class="exist"';} ?>>>></a>
</p>
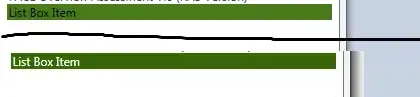