Create the HTTP Session manually before you call the login
method.
You can do this by calling the HttpServletRequest.getSession()
method, like this:
req.getSession(true); // Creates a new HTTP Session BEFORE the login.
req.login(user.getUsername(), user.getPassword());
Make sure the server is returning the JSESSIONID cookie in the response. After that the browser will take care of it and send it to the server without you having to worry about it or handling it yourself.
Response from the Login
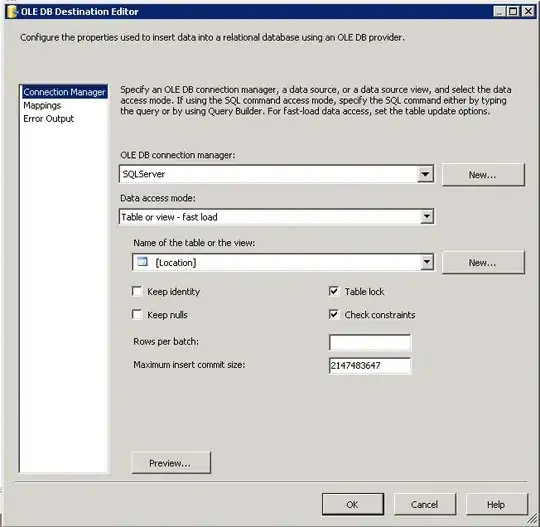
Request for further calls (service calls, check the login, logout, etc)
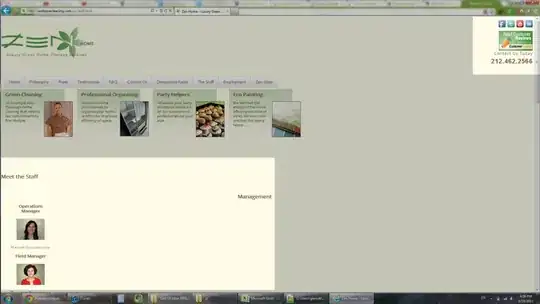
Update
Maybe you need to tell AngularJS to send the Cookies. For what I have read just now it seems that the default is not send cookies.
Try this post $http doesn't send cookie in Requests
Update 2
Cross-domain policies can be a pain but they need to exist in order to secure access to content in the web. The Java server is behaving normally, authentication is happening and the cookies are being shared. It is all good with the Java server since the beginning.
The problem is the same for any middleware technology when it come to cross-domain.
The browser is blocking your application because your client domain (where your JavaScript is running) is not allowed to access the Java server domain. The applications (your AngularJS and the Java Server) are executing in different domains, therefore JavaScript calls "under the hoods" are blocked by browsers. Old IE versions used to allow it in the past.
Example:
- JavaScript code is loaded from localhost or local file system
- Java Server is running at http://192.168.1.15:8080 (replace this for your IP or any address)
Maybe JSONP can save you:
JSONP allows a page to receive JSON data from a different domain by
adding a element to the page which loads a JSON response with
a callback from a different domain.
Cross-domain policy will verify: protocol, hostname and port, for both applications. If one is different, security takes place and the program fails.
More info here: Same-origin policy
There is also nothing wrong with your AngularJS. I ran across this situation in the past, and you can replicate it using plain Java Script (this is functional code that you can adapt to you problem):
function loadXMLDoc() {
var xmlhttp=new XMLHttpRequest();
xmlhttp.onreadystatechange=function() {
if (xmlhttp.readyState==4 && xmlhttp.status==200) {
document.getElementById("myDiv").innerHTML=xmlhttp.responseText;
}
}
xmlhttp.open("POST","http://192.168.9.117:8080/restful-login-web/rest/loginservice/login",true);
xmlhttp.setRequestHeader("Content-type","application/x-www-form-urlencoded");
xmlhttp.send("username=evandro&password=senha123");
}
The following error will show up in your browsers console:
XMLHttpRequest cannot load http://192.168.9.117:8080/restful-login-web/rest/loginservice/login. No 'Access-Control-Allow-Origin' header is present on the requested resource. Origin 'null' is therefore not allowed access.
It will also happen with GET calls.
You can allow your external application to use JavaScript to communicate with the Java server setting the Access-Control-Allow-Origin
somewhat like this (.header("Access-Control-Allow-Origin", "*")
):
return Response.status(Response.Status.OK).header("Access-Control-Allow-Origin", "*").type("text/plain").entity("Login successfull").build();
Warning: Replace the "*" for the domain in which your application client is running.
I suggest you to investigate on the cross-domain policy subject. If you can, maybe a simple solution is to run the client JavaScript inside Java container. Then both applications will be automatically in the same domain.
What I mean with that is that if you use a JSP/Servlet to publish your application client Java Script, you wouldn't have this problem. If you are developing the JavaScript in plain files, just wrap them inside the Java Web application. Since you will execute a Java Script code retrieved from "xyz.com" domain, the Java code running in the same "xyz.com" will be allowed to exchange information by the browser. This will work.
I am sorry, it took me more time than it should to realize you were doing an AJAX call to a different domain.
If you need to communicate different domains (maybe the server is an external service) and you can't find any help I suggest you to open another question specifically for the cross-domain issue. As I understand this problem is no longer associated with the Java server itself.