java.time
Use modern java.time classes rather than the troublesome legacy classes seen in the other Answer. Specifically, the ZonedDateTime
class.
By the way, those input string formats are terrible. Especially the use of EDT
, IST
, and such. Those are not true time zones. These are real time zone names. Those pseudo-zones are not standardized, and are not even unique! So the ZonedDateTime
class will take a guess as to what you might mean, but the results may be other than you intended. For example, is IST
Irish Standard Time or India Standard Time?
Instead, use only the standard ISO 8601 formats when exchanging date-time values as text.
I fixed your faulty input strings (Tuesday = 21, 22, and 23?).
List < String > inputs = List.of(
"Tue Oct 21 17:05:37 EDT 2014" ,
"Wed Oct 22 18:05:37 IST 2014" ,
"Thu Oct 23 19:05:37 EST 2014"
);
Define a formatting pattern to match your inputs.
DateTimeFormatter f = DateTimeFormatter.ofPattern( "EEE MMM dd HH:mm:ss z uuuu" ).withLocale( Locale.US );
Loop the inputs, attempting to parse each. Again, no guarantee as to the right time zone being guessed for your pseudo-zones.
for ( String input : inputs )
{
ZonedDateTime zdt = ZonedDateTime.parse( input , f );
System.out.println( "zdt.toString() = " + zdt );
}
When run.
zdt.toString() = 2014-10-21T17:05:37-04:00[America/New_York]
zdt.toString() = 2014-10-22T18:05:37Z[Atlantic/Reykjavik]
zdt.toString() = 2014-10-23T19:05:37-04:00[America/New_York]
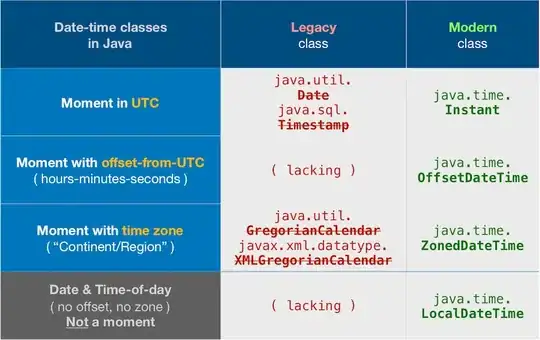
About java.time
The java.time framework is built into Java 8 and later. These classes supplant the troublesome old legacy date-time classes such as java.util.Date
, Calendar
, & SimpleDateFormat
.
To learn more, see the Oracle Tutorial. And search Stack Overflow for many examples and explanations. Specification is JSR 310.
The Joda-Time project, now in maintenance mode, advises migration to the java.time classes.
You may exchange java.time objects directly with your database. Use a JDBC driver compliant with JDBC 4.2 or later. No need for strings, no need for java.sql.*
classes.
Where to obtain the java.time classes?
The ThreeTen-Extra project extends java.time with additional classes. This project is a proving ground for possible future additions to java.time. You may find some useful classes here such as Interval
, YearWeek
, YearQuarter
, and more.