Here is my solution to your question:
Note: This code also finds overlapping sequences. Depending on whether you want to allow overlapping or not you will have to remove '?='
import re
class bcolors:
HEADER = '\033[95m'
OKBLUE = '\033[94m'
OKGREEN = '\033[92m'
WARNING = '\033[93m'
FAIL = '\033[91m'
ENDC = '\033[0m'
BOLD = '\033[1m'
UNDERLINE = '\033[4m'
my_file_m= '''TTCCATTCTCTACCCAGCCCCCACTCTGACCCCTTTACTCTGACCCCTTTATTGTCTACTCCTCAGAGCCCCCAGTCTGTATCCTTCTAACTTAGAAAGGGGATTATGGCTCAGGGTCCAACTCTGTGCTCAGAGCTTTCAACAACTACTCAGAAACACAAGATGCTGGGACAGTGACCTGGACTGTGGGCCTCTCATGCACCACCATCAAGGACTCAAATGGGCTTTCCGAATTCACTGGAGCCTCGAATGTCCATTCCTGAGTTCTGCAAAGGGAGAGTGGTCAGGTTGCCTCTGTCTCAGAATGAGGCTGGATAAGAT'''
pat = re.compile(r'(?=(TAA|AAT|TGA|TAG))') # Very important, if you do not need overlaps then remove '?='
matches = re.finditer(pat,my_file_m)
result1 = [int(match.start(1)) for match in matches] # find all the starting positions of the string
result2 = [range(x,x+3) for x in result1 ] # find all the positions of the characters (given that we search for patterns of length 3, can be modified for other lengths too )
result3 = set().union(*result2) # generate a union
for chari in range(len(my_file_m)): # colorize based on if it is in a sequence or not
if(chari in result3):
print bcolors.OKGREEN + my_file_m[chari] + bcolors.ENDC,
else:
print my_file_m[chari],
Cleaner:
import re
import sys
my_file_m= '''TAATTCCATTCTCTACCCAGCCCCCACTCTGACCCCTTTACTCTGACCCCTTTATTGTCTACTCCTCAGAGCCCCCAGTCTGTATCCTTCTAACTTAGAAAGGGGATTATGGCTCAGGGTCCAACTCTGTGCTCAGAGCTTTCAACAACTACTCAGAAACACAAGATGCTGGGACAGTGACCTGGACTGTGGGCCTCTCATGCACCACCATCAAGGACTCAAATGGGCTTTCCGAATTCACTGGAGCCTCGAATGTCCATTCCTGAGTTCTGCAAAGGGAGAGTGGTCAGGTTGCCTCTGTCTCAGAATGAGGCTGGATAAGAT'''
pat = re.compile(r'(?=(TAA|TGA|TAG))') # Very important, if you do not need overlaps then remove '?='
lettersToColor = set().union(*[range(m.start(1),m.start(1)+3) for m in re.finditer(pat, my_file_m)])
for chari in range(len(my_file_m)): # colorize based on if it is in a sequence or not
if(chari in lettersToColor):
sys.stdout.write('\033[92m' + my_file_m[chari] +'\033[0m')
else:
sys.stdout.write(my_file_m[chari])
Credit to : here and here
Output:
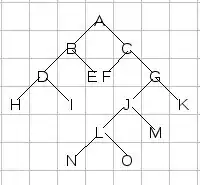