I don't want to use any layout menager since i want few circle images on my Panel.
Swing was designed at the core to use layout managers, it how it's update process works and how it deals with the difference in rendering pipelines across multiple platforms. Sure, you can try and do without it, but in the end, you'd simply reinvent the wheel as you try and compensate for all the little niggly issues that are created while trying to do without it.
The following simply uses a GridBagLayout
, which provides me with the ability to supply gridx/y positions to the components (and other nice things)
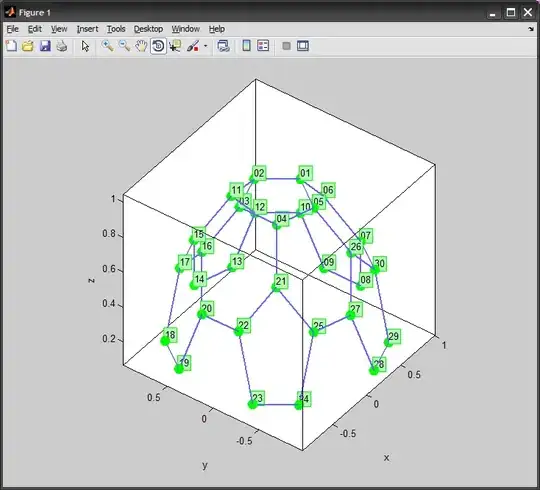
import java.awt.Color;
import java.awt.Dimension;
import java.awt.EventQueue;
import java.awt.Graphics;
import java.awt.Graphics2D;
import java.awt.GridBagConstraints;
import java.awt.GridBagLayout;
import java.awt.RenderingHints;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.JSeparator;
import javax.swing.UIManager;
import javax.swing.UnsupportedLookAndFeelException;
public class BinaryClock {
public static void main(String[] args) {
new BinaryClock();
}
public BinaryClock() {
EventQueue.invokeLater(new Runnable() {
@Override
public void run() {
try {
UIManager.setLookAndFeel(UIManager.getSystemLookAndFeelClassName());
} catch (ClassNotFoundException | InstantiationException | IllegalAccessException | UnsupportedLookAndFeelException ex) {
ex.printStackTrace();
}
JFrame frame = new JFrame("Testing");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.add(new TestPane());
frame.pack();
frame.setLocationRelativeTo(null);
frame.setVisible(true);
}
});
}
public class TestPane extends JPanel {
public TestPane() {
setLayout(new GridBagLayout());
GridBagConstraints gbc = new GridBagConstraints();
// Hour column
gbc.gridx = 0;
addColumn(2, gbc);
gbc.gridx = 1;
addColumn(4, gbc);
// Minute column
gbc.gridx = 3;
addColumn(3, gbc);
gbc.gridx = 4;
addColumn(4, gbc);
// Minute column
gbc.gridx = 6;
addColumn(3, gbc);
gbc.gridx = 7;
addColumn(4, gbc);
gbc.gridx = 2;
gbc.gridy = 0;
gbc.fill = GridBagConstraints.VERTICAL;
gbc.gridheight = GridBagConstraints.REMAINDER;
add(new JSeparator(JSeparator.VERTICAL), gbc);
gbc.gridx = 5;
add(new JSeparator(JSeparator.VERTICAL), gbc);
}
@Override
public Dimension getPreferredSize() {
return new Dimension(200, 200);
}
protected void addColumn(int count, GridBagConstraints gbc) {
for (int index = 0; index < count; index++) {
gbc.gridy = 3 - index;
add(new Dot(), gbc);
}
}
}
public class Dot extends JPanel {
@Override
public Dimension getPreferredSize() {
return new Dimension(100, 100);
}
@Override
protected void paintComponent(Graphics g) {
super.paintComponent(g);
Graphics2D g2d = (Graphics2D) g.create();
g2d.setRenderingHint(RenderingHints.KEY_ALPHA_INTERPOLATION, RenderingHints.VALUE_ALPHA_INTERPOLATION_QUALITY);
g2d.setRenderingHint(RenderingHints.KEY_ANTIALIASING, RenderingHints.VALUE_ANTIALIAS_ON);
g2d.setRenderingHint(RenderingHints.KEY_COLOR_RENDERING, RenderingHints.VALUE_COLOR_RENDER_QUALITY);
g2d.setRenderingHint(RenderingHints.KEY_DITHERING, RenderingHints.VALUE_DITHER_ENABLE);
g2d.setRenderingHint(RenderingHints.KEY_FRACTIONALMETRICS, RenderingHints.VALUE_FRACTIONALMETRICS_ON);
g2d.setRenderingHint(RenderingHints.KEY_INTERPOLATION, RenderingHints.VALUE_INTERPOLATION_BILINEAR);
g2d.setRenderingHint(RenderingHints.KEY_RENDERING, RenderingHints.VALUE_RENDER_QUALITY);
g2d.setRenderingHint(RenderingHints.KEY_STROKE_CONTROL, RenderingHints.VALUE_STROKE_PURE);
g2d.setColor(Color.DARK_GRAY);
g2d.fillOval(0, 0, getWidth() - 1, getHeight() - 1);
g2d.dispose();
}
}
}
This now frees you up to worry about how to make the clock update without need to continuously try and fix issues in the layout