how many calls will be stored in stack for the recursive method below and why?
With an input of 50, the max amount of stack frames at any given moment, including the initial call to fib
, will be 50. (So there will be at most 49 recursive calls stored on the stack at any given moment).
The reason there can be at most 50 stack frames is:
The initial call to fib(50)
is 1 stack frame.
Then with this statement, return fib( n - 1 ) + fib( n - 2 );
the recursive call to fib
on the left will execute first, putting fib(49)
on the stack. We'll hit the return line again putting fib(48)
on the stack. This will repeat until fib(1)
is on the stack hitting the return 1;
statement. This will return to fib(2)
and allow the right recursive call to execute in the statement return fib( n - 1 ) + fib( n - 2 );
.
To make long stories short, ALL the left recursive calls execute before even 1 right recursive call does. So it's easy to see you'll have fib(50)
, fib(49)
, ..., and finally fib(1)
for the last recursive call, leading to a maximum of 50 stack frames when executing fib(50);
.
When is something stored in stack exactly?
When you call a function, a stack frame is allocated, and after the function returns, the stack frame is deallocated. Even the function call for main
is stored on the stack. However, stack frames may be optimized away, but that is a more advanced topic.
See this question I asked when I was a wee lad if you'd like to know more about stack frames being optimized away:
Does a stack frame really get pushed onto the stack when a function is called?
Additional Edit:
If you'd like to understand how the recursion is happening with a visual, place your finger at the top of the root, and trace around the outside of the tree going down the left side first:
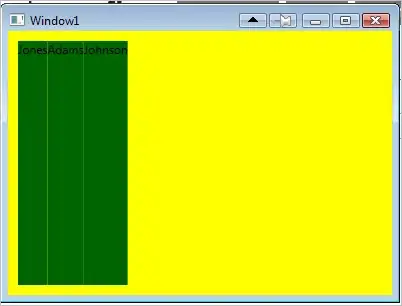