There's couple options. Maybe the easiest one is to wrap the values you want to databind to screen and call ToString for them. For example, if you have:
public decimal Value
{
get { return this.value; }
set
{
if (value == this.value) return;
this.value = value;
OnPropertyChanged();
}
}
Wrap it inside your ViewModel like this:
public decimal Value
{
get { return this.value; }
set
{
if (value == this.value) return;
this.value = value;
OnPropertyChanged("ValueString");
}
}
public string ValueString
{
get { return this.value.ToString(CultureInfo.CurrentCulture); }
}
And bind your UI against this new property:
<TextBlock x:Name="Result" Text="{Binding ValueString}" Grid.Row="0"/>
This way you will automatically get the formatting based on your computer's culture settings:
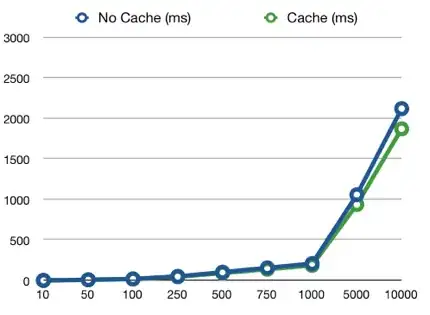
Another alternative is to use the method presented in here: https://stackoverflow.com/a/19796279/66988
So you need a custom Binding class:
public class CultureAwareBinding : Binding
{
public CultureAwareBinding(string path)
: base(path)
{
ConverterCulture = CultureInfo.CurrentCulture;
}
}
And then you have to use that in your XAML:
<TextBlock x:Name="Result" Text="{wpfApplication9:CultureAwareBinding Value}" Grid.Row="0"/>
After which you should see the desired output:
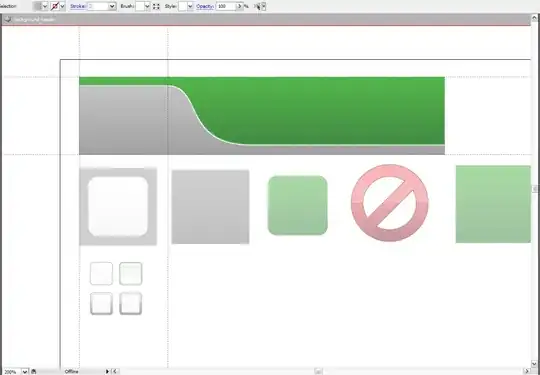