I’m adding to the correct Answer by Ole V.V.
JDBC 4.2
In particular - I am going to be working mainly with JDBC.
JDBC 4.2 added support for exchanging java.time objects with the database. See the PreparedStatement::setObject
and ResultSet::getObject
methods.
ZoneId z = ZoneId.of( "Africa/Tunis" ) ;
LocalDate today = LocalDate.now( z ) ;
myPreparedStatement.setObject( … , today ) ;
Retrieval.
LocalDate ld = myResultSet.getObject( … , LocalDate.class ) ;
For reasons that escape me, the JDBC spec does not require support for the two most commonly used classes: Instant
and ZonedDateTime
. Your database and JDBC driver may or may not add support for these.
If not, you can easily convert. Start with OffsetDateTime
, with support required in JDBC.
OffsetDateTime odt = myResultSet.getObject( … , OffsetDateTime.class ) ;
To see this moment through the wall-clock time used by people of a particular region (a time zone), apply a ZoneId
to get a ZonedDateTime
object.
ZoneId z = ZoneId.of( "Asia/Kolkata" ) ;
ZonedDateTime zdt = odt.atZoneSameInstant() ;
To adjust into UTC, extract an Instant
. An Instant
is always in UTC, by definition.
Instant instant = odt.toInstant() ;
You can convert the other way, to write to a database.
myPreparedStatement.setObject( … , zdt.toOffsetDateTime() ; // Converting from `ZonedDateTime` to `OffsetDateTime`. Same moment, same point on the timeline, different wall-clock time.
…and:
myPreparedStatement.setObject( … , instant.atOffset( ZoneOffset.UTC ) ) ; // Converting from `Instant` to `OffsetDateTime`. Same moment, same point on the timeline, and even the same offset. `OffsetDateTime` is a more flexible class with abilities such as (a) applying various offsets and (b) flexible formatting when generating text, while `Instant` is meant to be a more basic building-block class.
Notice the naming convention used in java.time: at
, from
, to
, with
, and so on.
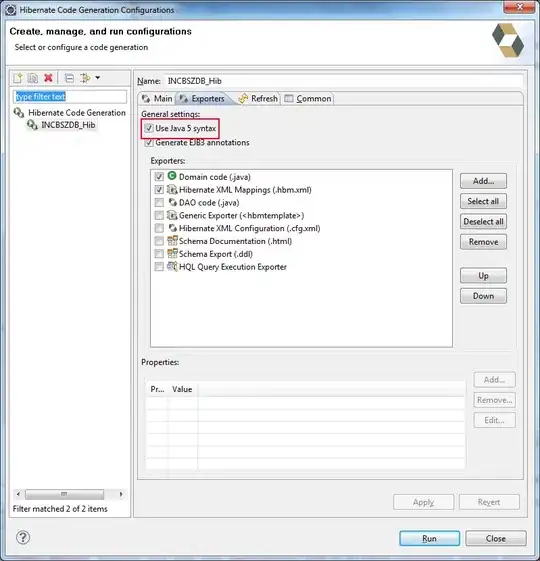
About java.time
The java.time framework is built into Java 8 and later. These classes supplant the troublesome old legacy date-time classes such as java.util.Date
, Calendar
, & SimpleDateFormat
.
To learn more, see the Oracle Tutorial. And search Stack Overflow for many examples and explanations. Specification is JSR 310.
The Joda-Time project, now in maintenance mode, advises migration to the java.time classes.
You may exchange java.time objects directly with your database. Use a JDBC driver compliant with JDBC 4.2 or later. No need for strings, no need for java.sql.*
classes.
Where to obtain the java.time classes?
The ThreeTen-Extra project extends java.time with additional classes. This project is a proving ground for possible future additions to java.time. You may find some useful classes here such as Interval
, YearWeek
, YearQuarter
, and more.