Expanding on Drew's answer here is an example of a Radial Gradient Brush using a PathGradientBrush
with a set of four named colors. To show the sphere in different colors it would be best to create the color array in code..
private void panel1_Paint(object sender, PaintEventArgs e)
{
Rectangle bounds = panel1.ClientRectangle;
using (var ellipsePath = new GraphicsPath())
{
ellipsePath.AddEllipse(bounds);
using (var brush = new PathGradientBrush(ellipsePath))
{
// set the highlight point:
brush.CenterPoint = new PointF(bounds.Width/3f, bounds.Height/4f);
ColorBlend cb = new ColorBlend(4);
cb.Colors = new Color[]
{ Color.DarkRed, Color.Firebrick, Color.IndianRed, Color.PeachPuff };
cb.Positions = new float[] { 0f, 0.3f, 0.6f, 1f };
brush.InterpolationColors = cb;
brush.FocusScales = new PointF(0, 0);
e.Graphics.FillRectangle(brush, bounds);
}
}
}
This results in a circle that looks like a 3d-sphere..
Update: In fact the (basically) same code that draws the sphere can be used to draw a nice shadow. Here are the changes: choose a location and size that suits your needs, use an array of 3 colors and use shades of black with alpha values of maybe: 0,96,192 with positions of 0f, 0.3f, 1f . This way the shadow will be semitransparent all over and fading out at the edges.. I add an example with a wooden background so you can see how the shadow blends..
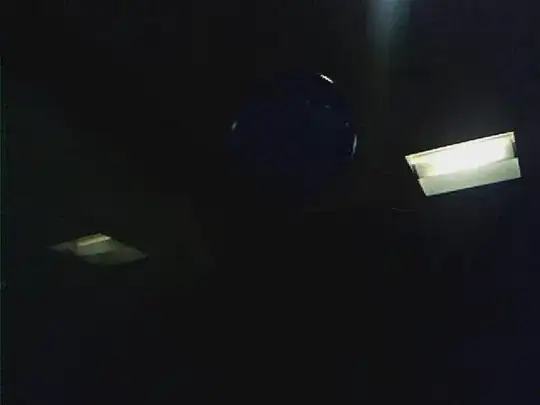
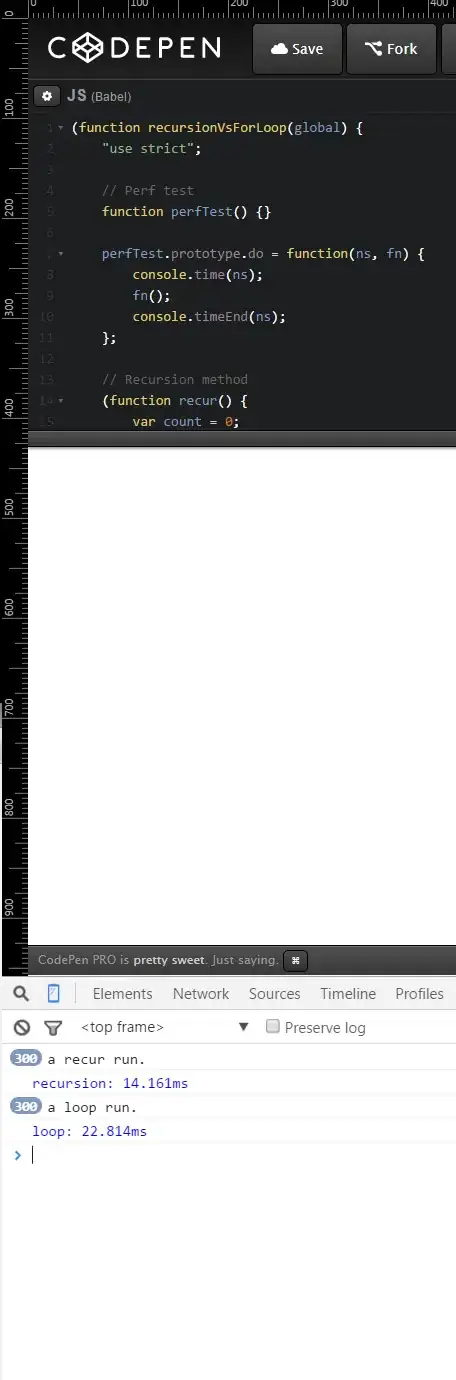