This is easily achievable with a custom drawable like this:
<?xml version="1.0" encoding="utf-8"?>
<shape xmlns:android="http://schemas.android.com/apk/res/android"
android:innerRadius="0dp"
android:shape="rectangle"
android:thicknessRatio="1.9"
android:useLevel="false">
<solid android:color="@android:color/transparent"/>
<stroke
android:width="125dp"
android:color="#CC000000"/>
</shape>
When you overlay that drawable on top of an image the results would looks something like this:
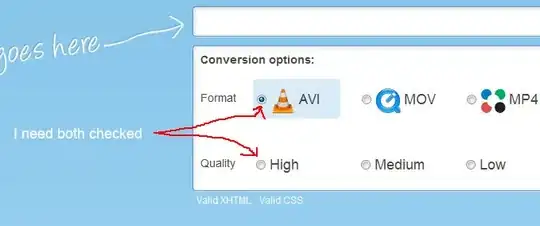
By creating that drawable dynamically in code you can adjust it at runtime. For example:
ShapeDrawable drawable = new ShapeDrawable(new RectShape());
drawable.getPaint().setColor(0xCC000000);
drawable.getPaint().setStyle(Paint.Style.STROKE);
drawable.getPaint().setStrokeWidth(dpToPixel(125.0f));
someView.setBackground(drawable);
You can influence the shape of the hole in the middle by combining multiple layers in a LayerDrawable
.
You can also influence the general shape of the hole by setting the android:shape
tag of the <shape />
element in the custom drawable.