The answer is very simple: don't use absolute layout, use a real LayoutManager
. In this case, it seems that BorderLayout
will do the job just fine.
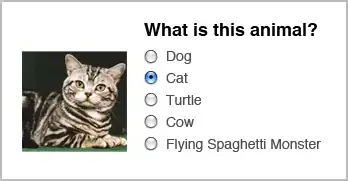
See this example:
import java.awt.BorderLayout;
import java.awt.Color;
import java.awt.Container;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JOptionPane;
import javax.swing.SwingUtilities;
public class TestLayout {
protected void initUI() {
JFrame frame = new JFrame("test");
Container cp = frame.getContentPane();
cp.setLayout(new BorderLayout());
cp.add(createColoredButton(Color.BLACK, Color.MAGENTA, "Hello World 1"), BorderLayout.NORTH);
cp.add(createColoredButton(Color.BLACK, Color.GREEN, "Hello World 2"), BorderLayout.EAST);
cp.add(createColoredButton(Color.WHITE, Color.BLUE, "Hello World 3"), BorderLayout.CENTER);
// frame.pack();
frame.setSize(600, 600);
frame.setVisible(true);
}
private JButton createColoredButton(Color fgColor, Color bgColor, final String text) {
final JButton button = new JButton(text);
button.setBorderPainted(false);
button.setFocusPainted(false);
button.setForeground(fgColor);
button.setBackground(bgColor);
button.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
JOptionPane.showMessageDialog(button, "You just clicked: " + text);
}
});
return button;
}
public static void main(String[] args) {
SwingUtilities.invokeLater(new Runnable() {
@Override
public void run() {
new TestLayout().initUI();
}
});
}
}